Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial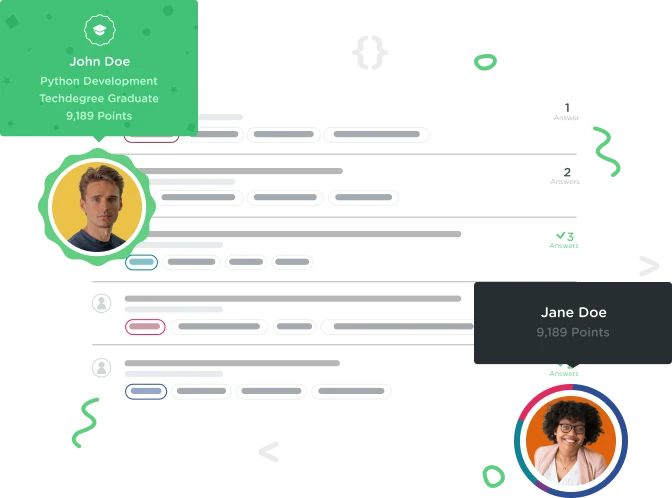

Marlon Jay Chua
4,281 Pointsminutes function
not sure if I am doing this the right way, there are too many variables on my code. Ca someone please assist. Thank you.
import datetime
def minutes(datetime1, datetime2):
seconds1 = datetime1.timedelta.total_seconds()
seconds2 = datetime2.timedelta.total_seconds()
dt1_round = round(seconds1/60)
dt2_round = round(seconds2/60)
diff = dt2_round - dt1_round
if diff >= 1:
return f'{dt2_round} {dt1_round}'
else:
return f'{dt1_round} {dt2_round}'
3 Answers
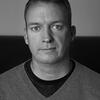
Andy Hughes
8,479 PointsMarlon Jay Chua - So the challenge asks you to find the difference between two dates in seconds. Then it's asking you to convert them to minutes and finally round them up. So in order:
- Create a timedelta variable that subtracts the older date (date1) from the newer date (date2) (remembering that the first date is always older. If you do it back to front, the challenge will tell you it got -1507 but was expecting 1507).
- All of the next steps can be included in one line of code:
- We can now use our timedelta, to find the seconds using timedelta.total_seconds()
- To find the minutes, we just add
/60
calculation after our total_seconds() - To round things up we just wrap our above code (steps 3-4) inside of the
round(your code goes here)
method. - It's less code and should pass the challenge.
If you want to cheat, my code is below :)
def minutes(date1, date2):
timedelta = date2 - date1
return round(timedelta.total_seconds()/60)
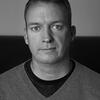
Andy Hughes
8,479 PointsIn the interest of keeping things simple and avoiding confusion. When this challenge says to use timedelta.total_seconds()
, you do not actually need to specify anything called timedelta
.
Having looked at the challenge again, it is really just saying "imagine your function takes two dates" (which subtracting one from the other gives a resulting timedelta automatically). So with this in mind, we can simplify my first answer to one line of code inside the function.
Instead of:
timedelta = date2 - date1
We can ignore the need to create a variable and just do the calculation as part of the return statement. So the conversion to minutes, rounding up and subtracting dates occur in the same line, as follows:
def minutes(date1, date2):
return round((date2 - date1).total_seconds()/60)
Hope that is clear and helps. :)

Marlon Jay Chua
4,281 PointsHi Andy! I didn't realize that you can write this code in 2 or 3 lines. The problem sounded complicated to me, but yes, your explanation is clear, and it worked. I did not know that you can use timedelta as a variable. Thank you!
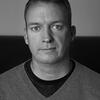
Andy Hughes
8,479 PointsMarlon Jay Chua - Just to be clear, the use of the word timedelta
in the challenge is not an actual timedelta, it is just a word. The actual timedelta is the output or result from subtracting the two dates.
Where the challenge says timedelta
you could in fact replace this with fish
, for example and the challenge would still pass. :)