Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial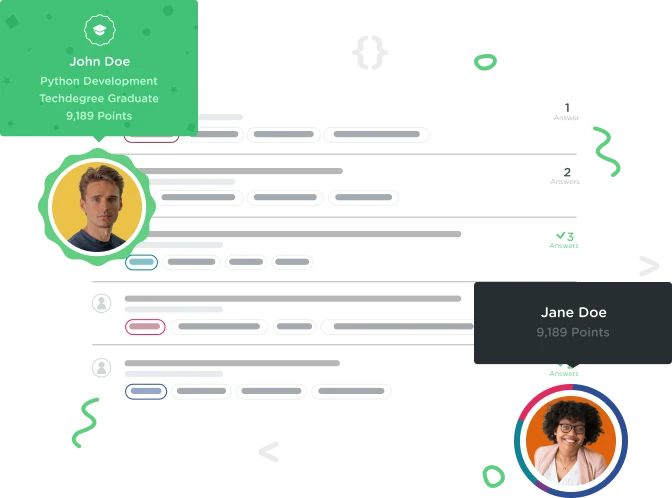

Benjamin Ong
5,786 PointsMissing return
I am not sure why there is a compiler error in my code, please help!
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if (obj instanceof String) {
result = (String) obj;
}
BlogPost store = Object;
if (obj instanceof BlogPost) {
store = (BlogPost) obj;
result = store.getTitle();
}
return result;
}
}
Edited markdown to reflect java rb
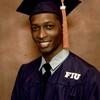
Dane Parchment
Treehouse Moderator 11,075 PointsPlease show us the code so that we can evaluate it and come up with a solution to help you!
1 Answer
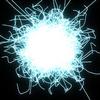
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHi Benjamin, I edited your answer to denote the java markdown, please see the style sheet if you have any questions.
As for why this isn't working, you need to return the results that your if clauses might catch inside the return block.
And when you're declaring your BlogPost object store, but that in your else if clause.
To do an else if clause you need to put else in front of your if, otherwise it will think that we have a brand new if statement.
I've copied the correct way to return the object when it's an instance of the String, and got you started with the BlogPost, I'll let you finish the rest. Shout back if you have trouble
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if (obj instanceof String) {
result = (String) obj;
return result;
}
else if (obj instanceof BlogPost) {
BlogPost store = (BlogPost) obj;
//need to add one or two more lines here depending how you want to solve this.
}
return result;
}
}

Benjamin Ong
5,786 PointsThanks Rob!! Managed to solve it. :D Got a little confused with how python works/
Evan Demaris
64,262 PointsEvan Demaris
64,262 PointsHi Benjamin,
Your code didn't attach; could you post it as well, so we can see what you've tried?