Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial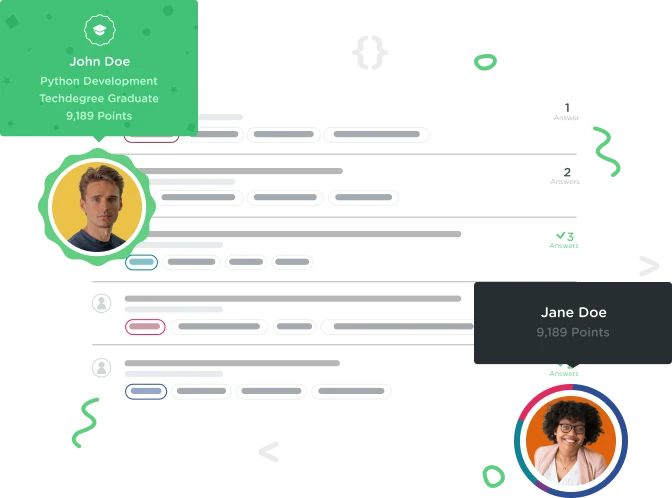

Joseph Bertino
Full Stack JavaScript Techdegree Student 14,652 PointsMisunderstanding how app.use() fires in sequence
I was under the impression that invocations of app.use()
get fired in sequence, according to how they are ordered in the .js files. But I noticed that if I moved the app.use()
which computes req.doubled
to AFTER the app.use()
that handles errors, the website still works flawlessly. What's going on here? My app.js is included below.
const express = require('express');
const path = require('path');
const bodyParser = require('body-parser');
const routes = require('./routes/index');
const app = express();
// view engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: false }));
app.use(express.static(path.join(__dirname, 'public')));
app.use('/', routes);
// catch 404 and forward to error handler
app.use((req, res, next) => {
const err = new Error('Not Found');
err.status = 404;
next(err);
});
app.use((req, res, next) => {
const number = parseFloat(req.body.number);
const result = number * 2;
req.doubled = result;
next();
});
// error handlers
// development error handler
// will print stacktrace
if (app.get('env') === 'development') {
app.use((err, req, res, next) => {
res.status(err.status || 500);
res.render('error', {
message: err.message,
error: err
});
});
}
// production error handler
// no stacktraces leaked to user
app.use((err, req, res, next) => {
res.status(err.status || 500);
res.render('error', {
message: err.message,
error: {}
});
});
module.exports = app;
3 Answers
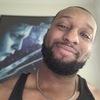
Brandon White
Full Stack JavaScript Techdegree Graduate 34,661 PointsHi Joseph,
Unless I’m missing something, there doesn’t seem to be anything present in the middleware function that adds the doubled property to the req object, that would break the error handling middleware. Now... if you moved the middleware function that adds the doubled property to the req object to after the error handling middleware then you shouldn’t have a doubled property on the req object, but unless the absence of that would break your error handling code, then your website should seem to work properly.
So you are correct in that the middleware is fired in sequence, but based on just the code you’ve presented switching the positions of the middleware functions you’ve mentioned would not break your site.
Is there something that you’re seeing that you believe would/should break your code?

Joseph Bertino
Full Stack JavaScript Techdegree Student 14,652 PointsHI Brandon,
The code that sets req.doubled
is in the middle of the snippet I posted.
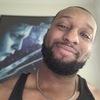
Brandon White
Full Stack JavaScript Techdegree Graduate 34,661 PointsCorrect. What I was saying is that there doesn’t appear to be anything happening in the code that sets req.doubled that is necessary in the next middleware function (ie the one handling errors).
Consider the following code:
app.use((req, res, next) => {
console.log(“This is message one!”)
next()
})
app.use((req, res, next) => {
console.log(“This is message two!”)
next()
})
The output from the above code would be: This is message one! This is message two!
But you could switch them around without any negative affects. Because there’s nothing happening in the first function that is required for the second function to perform properly.
That’s what I was getting at, if that makes sense. The middleware functions (ie. app.use()) do fire in order, but unless one function is dependent on the code manufactured in another function, then it won’t always matter the order that you place them in your code.

Joseph Bertino
Full Stack JavaScript Techdegree Student 14,652 PointsAhh I see. Thanks!