Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial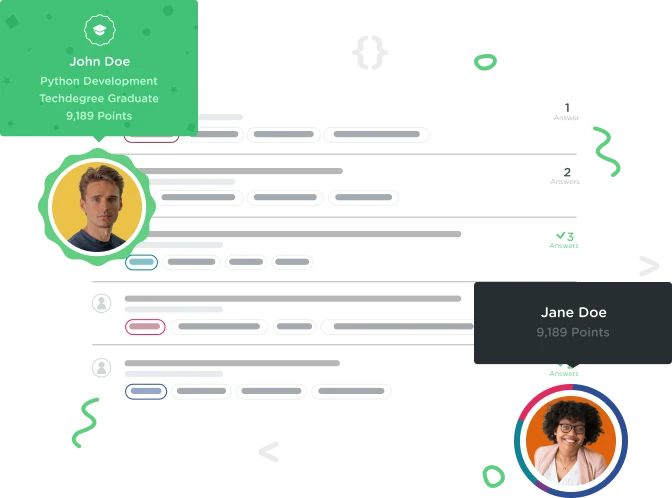

Patrick Eaton
3,860 PointsMisunderstanding or bug?
Hi!
I thought the attached code would be the correct solution for this challenge, but upon checking my work, I keep seeing the "Bummer: One or more of the buttons do not affect their previous sibling paragraphs" message. I've tried refreshing the preview page multiple times to click each button in turn, and each time the highlight
class appears to be applied to the previous paragraph correctly. Am I misunderstanding the correct approach?
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
list.previousElementSibling.className = 'highlight';
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
2 Answers
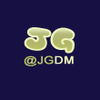
Jonathan Grieve
Treehouse Moderator 91,253 PointsI did a bit of digging on this for you and it looks like what's happening is the click event is only affecting the last list item in your code, So if you click "highlight" it will add the highlight class to the "things to learn" paragraph but it won't do the same for any of the others.
If you change the code you added so it calls previousElementSibling
on e.target
rather than list
it will add the same behaviour for each list item. It will then correctly highlight the elements preceding the button elements.

Patrick Eaton
3,860 PointsI see. Thank you for the link to the MDN documentation! Much of what the Basic JavaScript course has covered so far has been relatively easy to understand, but I think some additional reading in this area will do me good. ;-)
Patrick Eaton
3,860 PointsPatrick Eaton
3,860 PointsOh, I see. Thank you for looking into this for me, Jonathan! I can see that I misunderstood the instructions.
Incidentally, how does the JavaScript interpreter know what
e
represents in this scenario?Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsI couldn't tell you exactly how it knows or does its magic. But it works kind of the same way in practical terms that thw
hover
pseudo classes work in CSS in that they affect the instance of the selector that you are interacting with. That is often the functionality you want rather than selecting a specific element in code as you were previously doing withlist
.You can see this described if you have a look at the Mozilla documentation.
https://developer.mozilla.org/en-US/docs/Web/API/Event/target
"The target property of the Event interface is a reference to the object onto which the event was dispatched"