Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial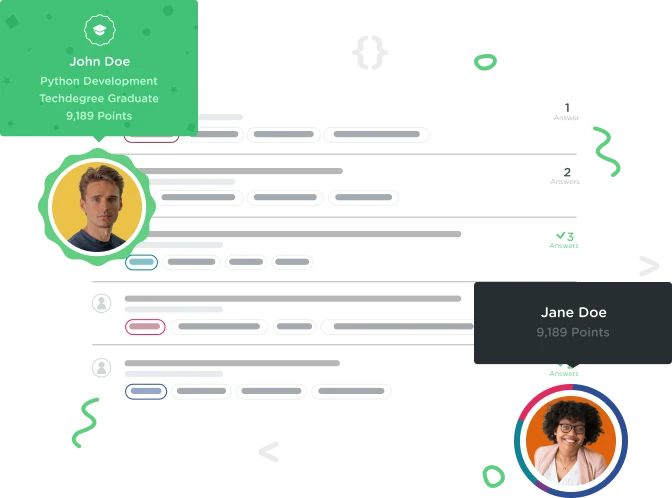
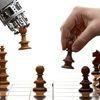
horus93
4,333 PointsMisunderstanding stringcases tuple challenge?
So, after a lot of tweaking I'm getting what I thought they wanted with the challenge here, a string of tuples for the different string case parameters it asked for.
- all uppercase
- all lowercase
- all titlecased
- reversed (though, I'm not sure if they want the words and letters backwards, or just the words in reverse order)
Anyway, it seems to work fine in my IDE, but in the challenge it says got the wrong strings for Uppercase, Lowrecase, Titlecased, and Reversed.
My IDE puts out this when I run it.
(('BOB,', 'FRANK,', 'TIM,', 'JOHN,', 'JILL'), ('bob,', 'frank,', 'tim,', 'john,', 'jill'), ('Bob,', 'Frank,', 'Tim,', 'John,', 'Jill'), ('jill', 'john,', 'tim,', 'frank,', 'bob,'))
def stringcases(str):
str = "bob, frank, tim, john, jill"
uc = str.upper()
uc_split = uc.split()
lc = str.lower()
lc_split = lc.split()
single_word = str.split()
tc = (' '.join((word.capitalize() for word in single_word)))
tc_split = tc.split()
reverse = tuple(single_word[::-1])
uppercase = tuple(uc_split[:])
lowercase = tuple(lc_split[:])
titlecase = tuple(tc_split[:])
return uppercase,lowercase,titlecase, reverse
print(stringcases(str))
4 Answers
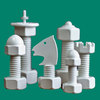
Steven Parker
231,275 PointsYou're close, but the challenge is expecting the output to be a tuple composed of four individual strings, but this code creates a tuple composed of four other tuples instead.
Hint: the input string won't need to be split. Each process can be done on the input string to generate a new string.
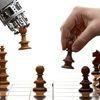
horus93
4,333 PointsYou're right, I did, and fixed that part by changing the reverse to this and ditching the split.
for l in str:
reverse = l + reverse
which put out this for the reverse portion
'llij nhoj mit knarf bob'
or, overall picture looked like this.
('BOB FRANK TIM JOHN JILL', 'bob frank tim john jill', 'Bob Frank Tim John Jill', 'llij nhoj mit knarf bob')
I thought maybe the naming conventions for the stuff inside was maybe throwing it off (because still getting the exact same wrong strings for everything message, but renaming stuff, and returning them all as separate entities at the end just produces the same results, and 'Bummer: Got the wrong string(s) for: Uppercase, Lowercase, Titlecase, Reversed'. :/
def stringcases(str):
str = "bob frank tim john jill"
reverse = ''
uppercase = str.upper()
lowercase = str.lower()
single_word = str.split()
titlecase = (' '.join((word.capitalize() for word in single_word)))
for l in str:
reverse = l + reverse
# convergence = uppercase, lowercase, titlecase, reverse
return uppercase, lowercase, titlecase, reverse
print(stringcases(str))
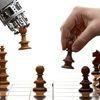
horus93
4,333 PointsOk, so I was banging my head against the wall until someone else pointed out something that should of been glaringly obvious, but was somehow blowing past me. Having the input string predefined in the code I was trying to put through into the challenge, which was preventing the challenge from properly putting a string through it, and explains why it was always giving me back all the strings coming back wrong. The output for the predefined string was right, but the challenge needed a variable input, and I wasn't allowing that.
def stringcases(str): #overwriting native function, big no no
str = "bob frank tim john jill" #overwriting the already overwritten function that isn't used in the code body above at all, dunno how that one flew past me, but still, this was the Achilles heel of the problem.
Also using str in the function was a bad idea because str is a native python class, so I was overwriting it (not to mention, that it wasn't being used after I overwrote it with the predefined string, so I was overwriting it for no reason).
Also found out my for / in loop was unnecessary, another oversight probably from forgetting some of the earlier coding lesson stuff from strings / lists. So instead of using a for / in loop to reverse the order (which works, but, easier to do with tools we'd already learned.)
reverse = input_string[::-1]
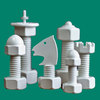
Steven Parker
231,275 PointsYou've successfully passed the challenge, right? You haven't marked the question solved yet.
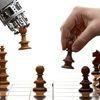
horus93
4,333 PointsYup, sorry, keep forgetting to do that :)
horus93
4,333 Pointshorus93
4,333 PointsSo, just to be clear then, the output format should be something like this then with 4 separate strings within the single tuple.
((BOB TIM), (bob tim), (Bob Tim), (tim bob)) or ('BOB TIM', 'bob tim', 'Bob Tim', 'tim bob')
I thought it'd be the second one, because within the tuple if they're wrapped in () again it indicates separate tuples from what i understand. [] is a list which isn't what we're looking for, so I'm not sure exactly what to shoot for.
I've trimmed the fat a bit, because that one up top has a lot of unnecessary excess code, but right now I'm basically getting back the second of those two examples
Output from that looks like this
('BOB FRANK TIM JOHN JILL', 'bob frank tim john jill', 'Bob Frank Tim John Jill', 'jill john tim frank bob')
Steven Parker
231,275 PointsSteven Parker
231,275 PointsEven closer now, but you forgot my hint about not needing "split". For the last one, the entire string should be reversed instead of placing the individual words in reverse order.