Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial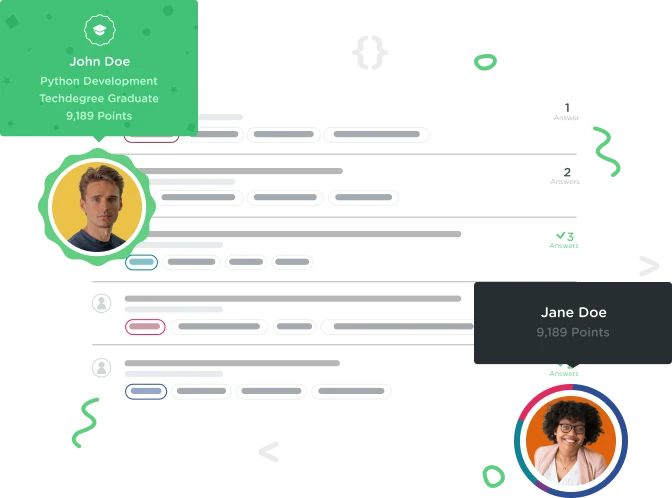
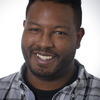
Paul Jackson
7,585 PointsMixed Semantics - value types mixed with reference types can change
In the example presented in the video:
import UIKit
struct Shape {
let shapeView: UIView
init(width: CGFloat, height: CGFloat, color: UIColor) {
let frame = CGRect(x: 0, y: 0, width: width, height: height)
shapeView = UIView(frame: frame)
shapeView.backgroundColor = color
}
}
let square = Shape(width: 100, height: 100, color: .redColor())
square.shapeView.backgroundColor = .blueColor()
Is it accurate to say that the color of the square is allowed to be modified because UIView
is class and therefore a reference type? And since the shapeView
defined as a constant is it means that the values can be changed, but you could not the reference to what shapeView
is pointing to in memory?
3 Answers
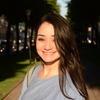
Tassia Castro
iOS Development Techdegree Student 9,170 PointsIs it accurate to say that the color of the square is allowed to be modified because UIView is class and therefore a reference type?
You are totally right. If UiView were a Struct, that means a value type, you wouldn’t be able to change the backgroundColor.
And since the shapeView defined as a constant is it means that the values can be changed, but you could not the reference to what shapeView is pointing to in memory?
Exactly. You are right. As shapeView was declared as a constant, you can only assign a UiView once. But ‘the values’ (backgroundColor) can be changed because UIView is a class and backgroundColor is a Var property inside UIView.

Boris Likhobabin
3,581 PointsA liitle off the topic but could you please explain why there is no SELF. in front of shapeView in initializer. Aren't we supposed to do that?
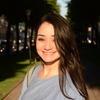
Tassia Castro
iOS Development Techdegree Student 9,170 PointsHey Boris,
You use self in front of a property during initialisation when the parameter of init() has the same name as the property of the class.
Check this simple example:
class car {
var name: String
var color: String
init(name: String, color: String) {
self.name = name
self.color = color
}
}
In this example if you don't use self the compiler will get confused. It will not know which one is the self property or the parameter received through the init().
So, going back to your question, as the init() does not have any parameter called shapeView, you don't need to use self and the compiler knows you mean self.shapeView.

Boris Likhobabin
3,581 PointsThanks a lot!
Paul Jackson
7,585 PointsPaul Jackson
7,585 PointsSweet! Thank you!