Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial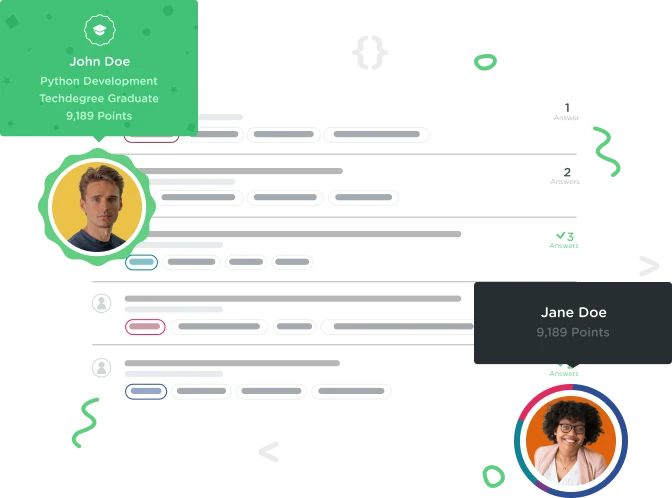
Giovanni Valdenegro
13,871 PointsModified if statement
So when Dave, is creating the xhr.readyState and status if statements he says he will modify his code within the if statement. He never explained why. He goes from this:
if (xhr.readyState === 4 && xhr.status ===200) {
// run code
}
to 2 if statements instead as below, what is the reason?? Why does he not use the &&.
if (xhr.readyState === 4) {
if(xhr.status === 200) {
document.getElementById('ajax').innerHTML = xhr.response;
}

Vance Rivera
18,322 PointsThe readyState indicates that the request to the server has finished. However it does not explicitly indicate if the request was successful or not. The status will indicate if the request was successful or not. The Status code has various helpful returns to indicate what happened. This will allow you to target a specific return from the status and handle it accordingly. Now to the point of just writing everything a single if statement, yes you can do that but for the sake of writing clean code and not repeating yourself you definitely would want to go with the second example you provided. If not you will end up with a ugly long evaluation in the nested if statement. Here is a reference to a list of status return values. http://www.w3schools.com/tags/ref_httpmessages.asp
Example of handling status:
if(xhr.readyState === 4) {
if(xhr.status === 200)
{
//handle success code here...
}
if(xhr.status === 401)
{
//handle unauthorized code here...
}
if(xhr.status === 404)
{
//handle not found code here...
}
}
Cheers!
3 Answers
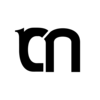
Colin Marshall
32,861 PointsI believe he moves it down from the original if statement to a nested if statement so that he can check for other status messages besides the 200 status, and display an error message of some sort if those other statuses were to arise.
if (xhr.readyState === 4) {
if (xhr.status === 200) {
document.getElementById('ajax').innerHTML = xhr.response;
} else if (xhr.status === 400) {
// file not found, do something
} else if (xhr.status === 500) {
// server problem, do something else
}
}
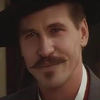
huckleberry
14,636 PointsI personally like denser code as long as it's not ridiculous and I actually find the first way easier to look at than more nested ifs. The less nesting the better imo.
if (xhr.readyState === 4 && xhr.status === 200) {
document.getElementById('ajax').innerHTML = xhr.response;
} else if (xhr.status === 400) {
// file not found, do something
} else if (xhr.status === 500) {
// server problem, do something else
}
}
Call me crazy but that just seems nicer to look at and easier for me to interpret.
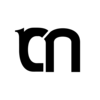
Colin Marshall
32,861 PointsThis does look cleaner, however I think there is an issue with setting it up this way. The readyState
should be 4 before the status is checked. With the way you have it set up, it will check for the status of 400 and 500 every time the readyState
changes, even if the readyState
is not 4. There are less "checks" performed if you nest the conditionals, which means less resources used. The difference is probably insignificant, but every little thing adds up.
If you are trying to avoid using nested conditionals, it should really look like this:
if (xhr.readyState === 4 && xhr.status === 200) {
document.getElementById('ajax').innerHTML = xhr.response;
} else if (xhr.readyState === 4 && xhr.status === 400) {
// file not found, do something
} else if (xhr.readyState === 4 && xhr.status === 500) {
// server problem, do something else
}
This way the conditional will always evaluate to false and skip checking the status if the readyState
is not 4.
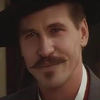
huckleberry
14,636 PointsAhhhhh very very good point! I sooo did not think of that. Thanks, Col!
Cheers,
Huck -
Giovanni Valdenegro
13,871 PointsHi Colin thanks for adding ``` to the js. He uses the else if statements for the other xhr.status. I think Vance is right it keeps the code clean by nesting the if statements. After he uses else if statements to check for other status.
Giovanni Valdenegro
13,871 PointsThanks guys for the support
Colin Marshall
32,861 PointsColin Marshall
32,861 PointsI fixed your code so it displays properly on the forum. You need to use 3 backticks followed by the language before and after the code like this:
```javascript
code goes here
```