Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial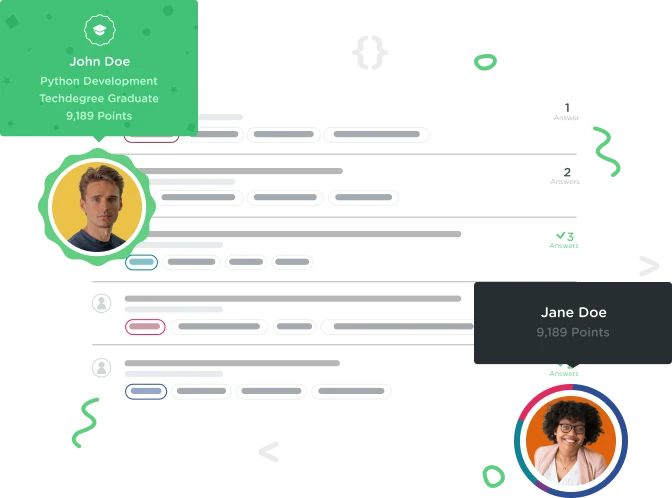

Raeed Sabree
10,664 Pointsmodify the $button click code so it now performs the redirect when the select is changed
what exactly do they want me to do because i tried changing select to .change
//Problem: It look gross in smaller browser widths and small devices
//Solution: To hide the text links and swap them out with a more appropriate navigation
//Create a select and append to #menu
var $select = $("<select></select>");
$("#menu").append($select);
//Cycle over menu links
$("#menu a").each(function(){
var $anchor = $(this);
//Create an option
var $option = $("<option></option>");
//Deal with selected options depending on current page
if($anchor.parent().hasClass("selected")) {
$option.prop("selected", true);
}
//option's value is the href
$option.val($anchor.attr("href"));
//option's text is the text of link
$option.text($anchor.text());
//append option to select
$select.append($option);
});
//Create button
var $button = $("<button>Go</button>");
//Bind click to button
$button.click(function(){
//Go to select's location
window.location = $select.val();
});
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<div id="menu">
<ul>
<li class="selected"><a href="index.html">Home</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
<li><a href="support.html">Support</a></li>
<li><a href="faqs.html">FAQs</a></li>
<li><a href="events.html">Events</a></li>
</ul>
</div>
<h1>Home</h1>
<p>This is the home page.</p>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
2 Answers
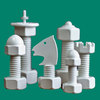
Steven Parker
230,274 PointsFor this step, you want to bind the handler that changes the location differently. Right now it's set up to be triggered by a click event on the button ($button). The challenge asks you to make it be triggered by a change event on the select ($select).
The comment that says //Bind click to button
doesn't have to be changed for the challenge, but if you wanted to make it accurate, after your other edits it should say something like //Bind change to select
.
I'm betting you can figure it out now without an explicit spoiler.
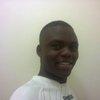
lan tapiwa mapfungautsi
22,170 Pointstraversing is changing an element inside the DOM (document object model ) to another element e.g a child to its parent element or vice versa
Raeed Sabree
10,664 PointsRaeed Sabree
10,664 Pointsokay so how do i modify the click button without changing the .click?
Steven Parker
230,274 PointsSteven Parker
230,274 PointsYou want to change the handler so instead of handling a button click, it handles a select change.
You won't use .click to establish the handler anymore.
Raeed Sabree
10,664 PointsRaeed Sabree
10,664 Pointsso what do i have to do in order to pass this challenge? what are they asking of me?
Steven Parker
230,274 PointsSteven Parker
230,274 PointsRight now, the handler that does the redirect is set up to be triggered by a click event on the button ($button). That's done on this line:
$button.click(function(){
The challenge is asking you to rewrite that line so instead of handling a button click, it handles a select change.
The title of this challenge is "Using change()" - that's also a hint.
Raeed Sabree
10,664 PointsRaeed Sabree
10,664 Pointsso add $button.change(function(){}) somewhere on the page?
Raeed Sabree
10,664 PointsRaeed Sabree
10,664 Pointsso add $button.change(function(){}) somewhere on the page?
Steven Parker
230,274 PointsSteven Parker
230,274 PointsThe button won't be the source of the change event. The select will.
And you aren't adding a new handler, you're changing the conditions that trigger the handler already there.
Raeed Sabree
10,664 PointsRaeed Sabree
10,664 Pointsso would it work better if instead of using $button.change(function(){} i use $button.select(function(){}
Steven Parker
230,274 PointsSteven Parker
230,274 PointsYou're going in the wrong direction. Select is not an event, it is an element, just like button is.
The whole idea is to change the handler so instead of handling a button click, it handles a select change.
At this point, it would probably be a very good idea to review the video just before the challenge, particularly from time index 0:45 to 2:50. You will see an example of this same thing being done.
Raeed Sabree
10,664 PointsRaeed Sabree
10,664 Pointsohh i had it right when i used change() i was just supossed to change the element from button to select
Raeed Sabree
10,664 PointsRaeed Sabree
10,664 Pointswhat is traversing?
Raeed Sabree
10,664 PointsRaeed Sabree
10,664 Pointswhat is traversing?
Marie Urbina
7,168 PointsMarie Urbina
7,168 PointsGreat hint, got it. Thanks! :)