Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial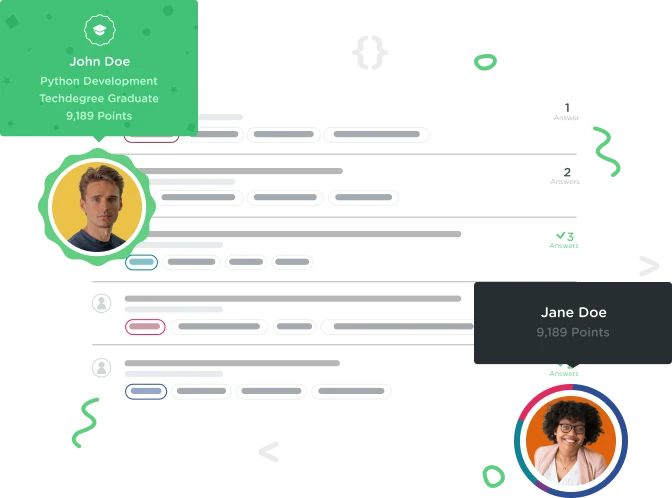

orange sky
Front End Web Development Techdegree Student 4,945 PointsModify the Car constructor
Hello,
I know this is not the correct answer, but some questions came along as I was trying to make sense of this code challenge.
1) In my code below, I don't understand why the currentGear in the increaseGear prototype does not increase, when I console.log( Civic.increaseGear.call(Civic));
2) if you run the code below, you will get:
" you have a Civic Its gear is 10"
It seems to me that the Javascript engine knows Car has these 2 values because of the constructor function, so why do I need to mention this.model and this.currentGear in the increaseGear() of the carPrototype. I would think passing the values (Civic, and 10) in the Object Civic and using this.model. and this.currentGear in the constructor method is enough. What is the intuition behind mentioning this.model and this.currentGear in the Construtor function and then in the prototype?
3) In the code below, to get it to print the model, and currentGear of my Object Civic, I noticed I could get it to print these 3 ways, but which is the most correct for a code that does not have an array:
console.log( Civic.increaseGear(Civic));
console.log( Civic.increaseGear.apply(Civic));
console.log( Civic.increaseGear.call(Civic));
4)
var carPrototype = {
model: "generic",
currentGear: 0,
increaseGear: function() {
return " you have a " + model +
' Its gear is ' + currentGear ++;
}
}
Civic = new Car ();
console.log( Civic.increaseGear.call(Civic));
When I adjust the code below, with the above statements, the web console does not print anything. I was expecting to see 'you have a generic model Its gear is 0' Since this Civic object does not have any values in its parameters. Why doesnt it output the values of the prototype (generic, 0)?
5) As for the real task of this assignment, I am not sure what I should do, can you please explain this code challenge.
thanks!!
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Objects</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Objects: Prototypes</h2>
<script>
var carPrototype = {
model: "generic",
currentGear: 0,
increaseGear: function(model, currentGear) {
return " you have a " + this.model +
' Its gear is ' + this.currentGear ++;
}
}
function Car(model, currentGear) {
this.model = model;
this.currentGear= currentGear;
}
Car.prototype = carPrototype;
Civic = new Car ("Civic", 10);
console.log( Civic.increaseGear.call(Civic));
</script>
</body>
</html>
3 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsThere's no reason to use call()
or apply()
on an object that has a method you're calling/applying. You are basically applying Civic
's own method on itself.
You can call the method like this: Civic.increaseGear()
. The method takes no parameters, so you don't need to pass the Civic
object as a parameter.
The call/apply mechanism is used to call functions as if they were methods on an object. If you have an object that doesn't have a method, by using call/apply, you can bind the value of this
inside a function to the object, thus making the function act like a method on that object.
For instance, if you were to create a new car object that wasn't prototype-linked to Car
, you could use the methods from the Civic
object on the newly created object:
var yaris = { // the yaris object has no methods, but it has a currentGear property
model: "Yaris",
currentGear: 0
};
console.log(yaris.currentGear); // 0
// so we can call/apply the method from Civic on yaris and get it to work and increase the currentGear property
Civic.increaseGear.call(yaris);
console.log(yaris.currentGear); // 1
The goal of this code challenge is much simpler, though. Task one asks you to modify the constructor function so it accepts a parameter and sets its model to the value passed in the parameter:
function Car(model) {
this.model = model;
}
The next task asks you to set the Car
's prototype to carPrototype
:
Car.prototype = carPrototype;
And finally, you need to modify the Car
's prototype so it has wheels
set to 4
:
Car.prototype.wheels = 4;
That's all there is to this challenge.

orange sky
Front End Web Development Techdegree Student 4,945 PointsThank you for explaining call/apply and showing me how call /apply can bind one Obj's method to another obj without using prototype.

orange sky
Front End Web Development Techdegree Student 4,945 PointsAs for the first task, I saw these methods in the prototype and I assumed I had to use them right after creating a constructor, and an instance with a value passed as its argument.
Ok, now I see, some of these tasks will have extra code in them, but I should simply focus on the instructions Thank you so much Dino!!!!
Farhat Jehan
7,268 PointsFarhat Jehan
7,268 PointsThanks a bunch :D