Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial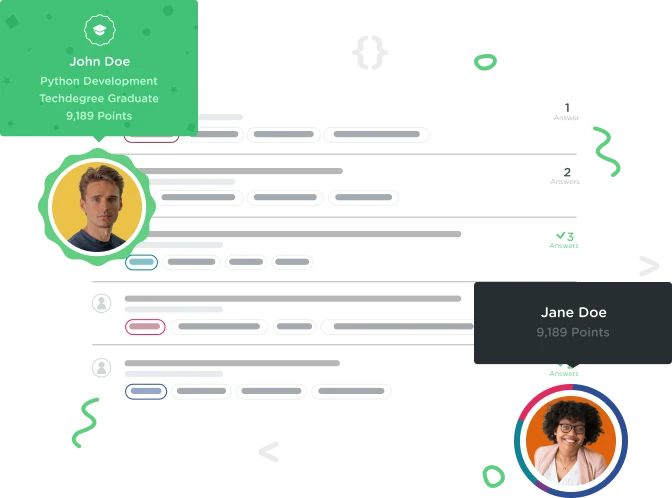
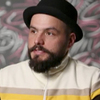
sergio verdeza
Courses Plus Student 10,765 PointsModify the Car's prototype to have 'wheels' set to 4. Hello, having trouble with this question? Help.......
var carPrototype = { model: "generic", currentGear: 0,
increaseGear: function() {
this.currentGear ++;
},
decreaseGear: function() {
this.currentGear--;
}
}
function Car (model) { this.model = model; }
Car.prototype = carPrototype;
Car.prototype.wheels = 4
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Objects</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Objects: Prototypes</h2>
<script>
var carPrototype = {
model: "generic",
currentGear: 0,
wheels: 4,
increaseGear: function() {
this.currentGear ++;
},
decreaseGear: function() {
this.currentGear--;
}
}
function Car(model) { this.model = model; }
Car.prototype.wheels = 4;
</script>
</body>
</html>
2 Answers
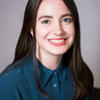
Madeleine Nyhagen
2,880 PointsI think you are just doing too much. I was successful using Car.prototype.wheels = 4; but did not add anything directly to the carPrototype variable. I believe my code looked like this:
var carPrototype = {
model: "generic",
currentGear: 0,
increaseGear: function() {
this.currentGear ++;
},
decreaseGear: function() {
this.currentGear--;
}
}
function Car(model) {
this.model = model;
}
Car.prototype = carPrototype;
Car.prototype.wheels = 4;

chris slaats
5,996 PointsFor this question you don't have to use Car.prototype.wheels = 4; because you have set the Car.prototype = carPrototype already. Then all the question is asking is of you is to change the Car's prototype which you have just set to carPorotypes variable wheels to 4 so it should look like this. Hope that helps
var carPrototype = {
model: "generic",
currentGear: 0,
wheels: 4,
increaseGear: function() {
this.currentGear ++;
},
decreaseGear: function() {
this.currentGear--;
}
}
function Car(model) {
this.model = model;
}
Car.prototype = carPrototype;