Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial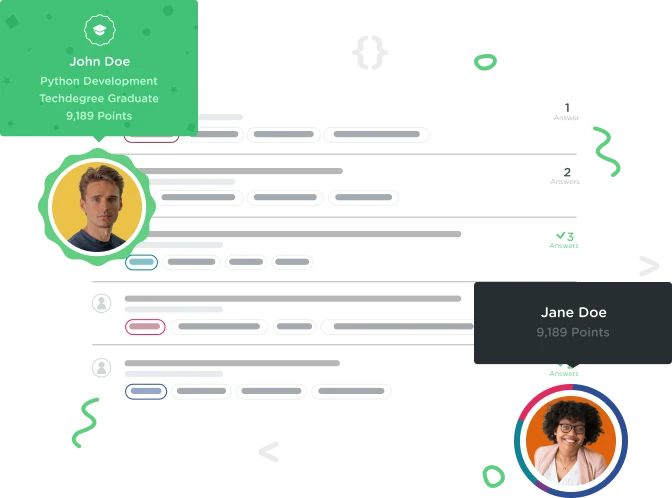
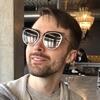
trottly
5,394 PointsModifying array that was declared with `const` keyword?
How are we able to modify an array (for example, using the array methods to add or remove elements) that has been declared with the const
keyword? Wouldn't this make the array immutable to begin with?
2 Answers
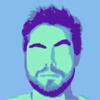
Cameron Childres
11,820 PointsHi Trottly,
The keyword const
can be a little misleading. From w3schools.com page on const:
It does NOT define a constant value. It defines a constant reference to a value.
Because of this, we cannot change constant primitive values, but we can change the properties of constant objects.
This means that we can change, add, or remove items within the referenced array -- but we can't change the overall reference.
This is possible:
const numbers = [1, 2, 3]
numbers[0] = 0
// array is now [0, 2, 3]
But this is not:
const numbers = [1, 2, 3]
numbers = [0, 2, 3]
// produces error
The first example changes the value of an item in the array, where the second example attempts to reassign the variable to a new array. I hope this helps! Let me know if you have any questions.

Busra Tokuc
7,444 PointsHi, You can check this article.
trottly
5,394 Pointstrottly
5,394 PointsIs there a way to make an array thats elements may also not be changed?
Cameron Childres
11,820 PointsCameron Childres
11,820 PointsYou can with Object.freeze():