Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial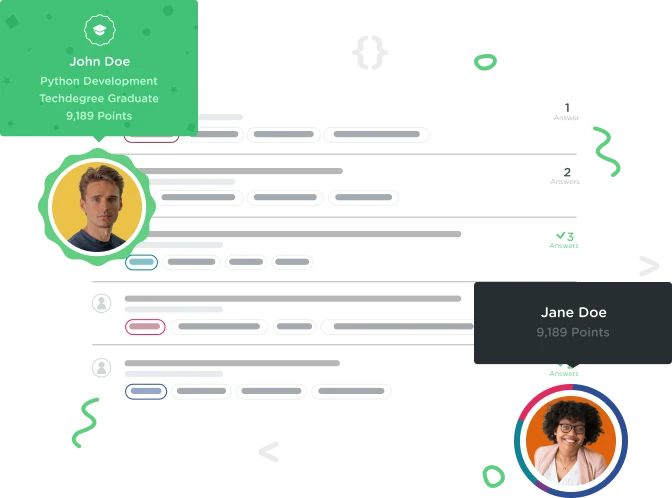

louiseretzlaff
8,325 PointsModifying Elements
Hello
I’m trying to set the text content of the a element as the input Value but using reassignment.
var inputId= document.getElementById('linkName');
var inputValue = inputId.value;
inputValue = document.getElementById('link').textContent;
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<label>Link Name:</label>
<input type="text" id="linkName">
<a id="link" href="https://teamtreehouse.com"></a>
</div>
<script src="app.js"></script>
</body>
</html>
2 Answers
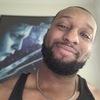
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsHI louiseretzlaff,
Great job on completing part one of the challenge.
If you look at the third line you've written you'll notice that you've called textContent on the anchor element with the id of link. Now all you have to do is set the value of the of the anchor elements textContent to something. In this case, what you want to set the value to is the inputValue variable declared on line two.
In addition, on the third line you're currently changing the value of what's stored in the inputValue declared on line two the the textContent of the anchor element with the id of link, which is nothing. So while you're setting the value of textContent for the anchor element with the id of link, you will also not store it to a variable (or at least you don't want to store it in the inputValue variable).
Keep at it coder!

Happy .
11,414 Pointshttps://developer.mozilla.org/en-US/docs/Web/API/Node/textContent
This stumped me for a while, but it's simpler than it looks.
You don't need to change the value of inputValue after it's been set, nor create a new variable, you need to GET the existing element('link') and SET textContent to inputValue
I hope that makes a bit more sense when trying to code your answer.
Melissa Ferguson
8,274 PointsMelissa Ferguson
8,274 PointsBrandon, I get what you are saying in the second par about not storing it in the variable but the directions say to set it to the variable inputValue and so did you in the last sentence of the first par. so can you be more clear please bc I am stuck on this as well.
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsWhat you want to do is grab the anchor element with an id of 'link' the same way you grabbed the input element with an id of 'linkName', and you want to set the textContent of the anchor element with an id of 'link' (since it currently does not have any text content) to be the value of inputValue (which we're saying is Louise's Link).
Challenge 1: Select the <input> element with the ID linkName and store its value in the variable inputValue. (For a refresher on getting the value of a text input, watch this video from the previous section.)
You've already completed challenge 1 with the first two lines of your code.
Challenge 2: Next, set the text content of the <a> element with the ID link to the value stored in inputValue.
The second challenge doesn't ask you to store the text content of the <a> element with the ID link to inputValue, it asks that you set the text content of the <a> element with the ID link to the value [already] stored in inputValue.
Does this make sense? I know it can be a lot to take in.
Melissa Ferguson
8,274 PointsMelissa Ferguson
8,274 PointsI appreciate you breaking it down Brandon, however, I still lost. I'm sorry. I see how it would replace the value bc you are using the same variable to hold a different value. Am I understanding this correctly? I've looked text content online and went over the treamhouse video again and I still don't get why my answer is not working? This is what I came up with:
var inputValue=document.getElementById('linkName').value;//first answer inputValue.textContent=document.getElementById('link');//I am calling that value to using text content and setting it to the id link which is attached to the element 'a'. Please tell me what I am missing?
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsFirst off, you have no need to apologize. You’re asking questions, and that’s a good thing.
Secondly, great job refactoring your code so that you grabbed the <input> with ID of linkName and its value in one statement.
Now, in order to grab the <a> with an ID of link, you’d write...
document.getElementById(‘link’)
,and if you wanted to GET the text content of the <a> with an ID of link, you’d write...
document.getElementById(‘link’).textContent
,and so if you wanted to SET the text content of the <a> with an ID of link, you’d write the code to GET the text content, but you’d SET it equal to the text you want it to be. So say you wanted the text content of the <a> with an ID of link to be Melissa’s Link...
You’d write...
document.getElementById(‘link’).textContent = “Melissa’s Link”
,And since that’s how you would set the text content of an element, how would you alter that line of code to set the text content to be equal to the value stored in the variable inputValue?
P.S. Yes, you understood the first part correctly. :), and sorry for just now realizing you are not the same person who posted the question asking for help to begin with. lol
Melissa Ferguson
8,274 PointsMelissa Ferguson
8,274 PointsOk I got it now, Brandon! that example helped. I had to set it to the variable but not change the value of the variable. so my answer was: document.getElementById('link').textContent=(inputValue);
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsYes. That’s exactly right, Melissa.
Now... you actually do not need to wrap inputValue in parentheses.
You could write it
document.getElementById(‘link’).textContent = inputValue
But adding the parentheses doesn’t alter the functionality. Good stuff.
Melissa Ferguson
8,274 PointsMelissa Ferguson
8,274 PointsGood to know! Thanks for all your help and patience!