Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial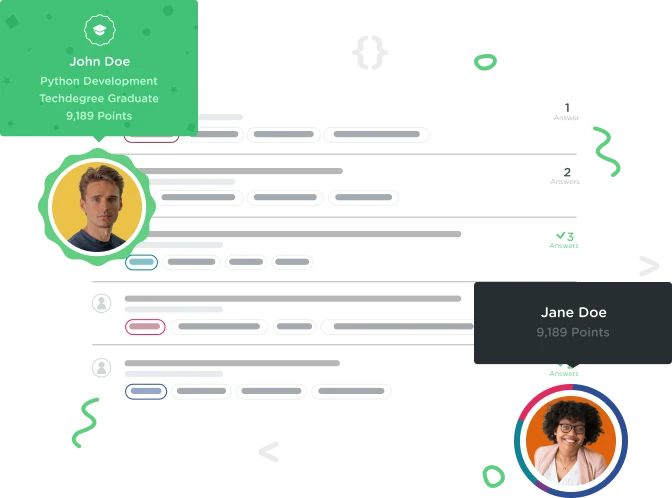

Gary Gibson
5,011 PointsModifying Number Guessing Game To Include A Tally Of Wins And Losses
I think I have to add empty lists called total_wins and total_losses at the top. But then I'm not sure where to put total_wins.append(player_guess) and total_losses.append(player_guess) so that the numbers actually tally. The way I've done it so far doesn't let the tally get higher than 1.
import random
def game():
the_magic_number = random.randint(1,20)
guesses = []
while len(guesses) < 5:
print(the_magic_number)
player_guess = (input("Can you guess the right number between 1 and 20 in five tries? "))
try:
player_guess = int(player_guess)
except ValueError:
print("That '{}' is not a number!".format(player_guess))
else:
if player_guess == the_magic_number:
print("You guessed it! {} was the number!".format(player_guess))
break
elif player_guess > 20:
print("{} is greater than 20. Please guess a number less than 20.".format(player_guess))
elif player_guess < the_magic_number:
print("{} is too low!".format(player_guess))
elif player_guess > the_magic_number:
print("{} is too high!".format(player_guess))
else:
print("Sorry. That's not right. Try again.")
guesses.append(player_guess)
if len(guesses) < 5:
print("{} guesses left!".format(5 - len(guesses)))
else:
print("You're out of guesses!")
else:
print("You didn't get it. My number was {}.".format(the_magic_number))
play_again = input("Do you want to play again? Y/n ")
if play_again.lower() == 'n':
print("Okay, see you later!")
else:
print("")
game()
game()
Note I have the program spit the correct answer out immediately so I can quickly test if the wins are being tallied.
3 Answers
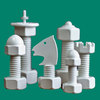
Steven Parker
230,274 PointsBe sure to put your code between lines with: "```py" and "```" to insure your indentation is preserved!
But I believe I reconstructed it correctly, and it worked as expected, counting down the tries with each input:
treehouse:~/workspace$ python tally.py
17
Can you guess the right number between 1 and 20 in five tries? 10
10 is too low!
4 guesses left!
17
Can you guess the right number between 1 and 20 in five tries? 15
15 is too low!
3 guesses left!
17
Can you guess the right number between 1 and 20 in five tries? 18
18 is too high!
2 guesses left!
17
Can you guess the right number between 1 and 20 in five tries? 16
16 is too low!
1 guesses left!
17
Can you guess the right number between 1 and 20 in five tries? 17
You guessed it! 17 was the number!
Do you want to play again? Y/n
Did it work differently for you?
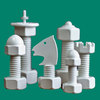
Steven Parker
230,274 PointsSimple counters could do that. So where you win:
if player_guess == the_magic_number:
print("You guessed it! {} was the number!".format(player_guess))
win += 1
break
And where you lose:
print("You didn't get it. My number was {}.".format(the_magic_number))
lose += 1
And since game calls itself to replay, you could just pass them as arguments:
if play_again.lower() == 'n':
print("Okay, see you later!")
print("You won {} games and lost {}.".format(win,lose))
else:
print("")
game(win, lose)
game(0, 0)

Gary Gibson
5,011 PointsThanks, I'd added counters, but no matter where I placed them, they wouldn't tally past 1.

Gary Gibson
5,011 PointsStill doesn't work. I'd placed counters for win and lose at the same places you suggested. But the script only records the first result (one win or one loss), then fails to tally any after that. The results thereafter are forever "wins: 1, losses: 0" or "wins: 0, losses: 1." The numbers never change after these initial results no matter how many times I play the game.
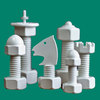
Steven Parker
230,274 PointsDid you forget to change the definition for game? I didn't show it explicitly in the previous set of changes, but you should have gotten an error if you left it out.
Well, maybe you had some typo or other issue adding in the changes. So here's your entire program with my changes from above (plus the definition), you can cut-n-paste it to the workspace to see how it tallies correctly:
import random
def game(win, lose):
the_magic_number = random.randint(1,20)
guesses = []
while len(guesses) < 5:
print(the_magic_number)
player_guess = (input("Can you guess the right number between 1 and 20 in five tries? "))
try:
player_guess = int(player_guess)
except ValueError:
print("That '{}' is not a number!".format(player_guess))
else:
if player_guess == the_magic_number:
print("You guessed it! {} was the number!".format(player_guess))
win += 1
break
elif player_guess > 20:
print("{} is greater than 20. Please guess a number less than 20.".format(player_guess))
elif player_guess < the_magic_number:
print("{} is too low!".format(player_guess))
elif player_guess > the_magic_number:
print("{} is too high!".format(player_guess))
else:
print("Sorry. That's not right. Try again.")
guesses.append(player_guess)
if len(guesses) < 5:
print("{} guesses left!".format(5 - len(guesses)))
else:
print("You're out of guesses!")
else:
print("You didn't get it. My number was {}.".format(the_magic_number))
lose += 1
play_again = input("Do you want to play again? Y/n ")
if play_again.lower() == 'n':
print("Okay, see you later!")
print("You won {} games and lost {}.".format(win,lose))
else:
print("")
game(win, lose)
game(0, 0)
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsIt works that way. I was trying to add a different count: one each for a tally of wins and losses.
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsI wanted to add something that tallied how many games were won and how many were lost.