Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial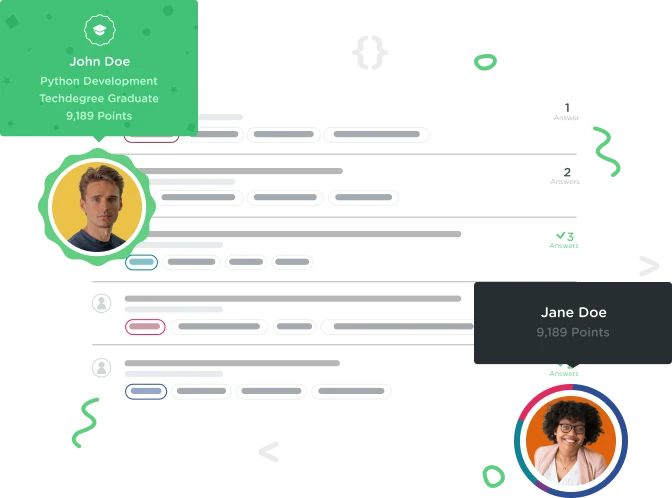
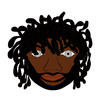
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsModular question.
Hi guys, I'm doing some practice in javaScript and I came upon a question that is giving a headache.
Q: Write a code that will print all the odd values from 1 to 600 using the while loop.(Hint: use the modulus operator)
Can anyone show me a solution to this?
The modulus from what I understand returns the remainder when two numbers are divided together. But how am I supposed to use it with the while loop to print all the odd values.
var odd = 1;
while (odd % 2 ==1) {
document.write(odd + " ");
odd++;
}
Is all I could come up with. How will I tell it to only print up to 600? Steven Parker
1 Answer
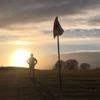
Stuart Wright
41,118 PointsI don't think you can use the modulus operator in your while statement, as it will break as soon as it hits the first even number (2). You could instead do something like this:
var odd = 1;
while (odd < 600) {
if (odd % 2 === 1) {
document.write(odd + " ");
}
odd ++;
}
Personally I would do this without the modulus operator:
var odd = 1;
while (odd < 600) {
document.write(odd + " ");
odd += 2;
}
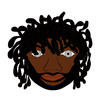
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsStuart Wright youβre right, it did break after printing 1. But itβs a question from a JavaScript book Iβm reading and thatβs how they asked to do it. I donβt know why. The book is titled βJavaScript projects for kidsβ
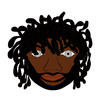
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsOk guys. Steven Parker and Stuart Wright I'm taking your advice and solution. I think you're right and it's just the way I'm thinking that needs to be adjusted. I think my thinking is too rigid and confined. I assumed because we were learning about the "while-loop" that the question meant only use the while loop and the modulus, however a few lessons before, we did learn about the conditional statement and the question didn't say we couldn't use all we've learned so far, which is exactly what we should be doing. It simply said "write a code".
I must admit though. When I saw the (Hint: use the modulus operator) I assumed they meant that it's the main solution to the problem so I focused on only using the modulus operator together with the while loop to solve the problem, never thinking about, or telling myself not to use any other thing we learned so far.
However they did require we used the while loop to solve this particular problem.
So thanks again guys. Big help.
Steven Parker
229,783 PointsSteven Parker
229,783 PointsI don't think the instructions meant you should use the remainder as part of the "while" condition. But it can be handy inside the loop body for printing out only the odd numbers as shown in Stuart's first example.