Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial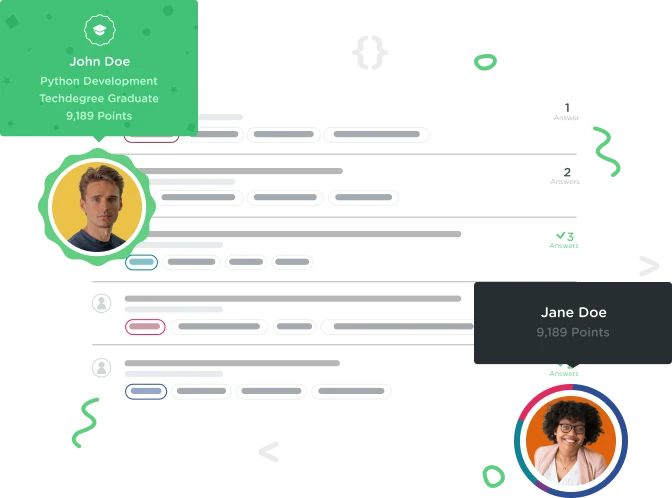

Mateusz Konach
330 PointsModule is overwriting
var awesomeNewModule = (function() {
var exports = {
one: 1,
two: 2
}
return exports;
}());
var awesomeNewModule = (function(exports){
var exports = {
foo: 5,
bar: 10
};
exports.helloMars = function() {
console.log("Hello Mars!");
};
exports.goodbye = function() {
console.log("Goodbye!");
};
return exports
}(awesomeNewModule || {}));
When I'm calling the awesomeNewModule, "one" and "two" variables doesn't exist anymore, how to fix that?
1 Answer
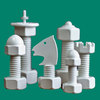
Steven Parker
231,169 PointsThe variables need to have different names.
When you create the second "awesomeNewModule", it takes over the one that was already there. If you don't want that to happen, just give one of them a different name.
Mateusz Konach
330 PointsMateusz Konach
330 PointsYeah, I thought I understand that but in video (11:38) it was said that if module with that name exists, it will extend it. Now I'm wondering how could I add these variables without overwriting existing one?
I could do it this way, but is it achieavable using object literal?
Steven Parker
231,169 PointsSteven Parker
231,169 PointsI get it now. I was confused because normally you would not have both of these in the same file.
But I think there may be an error in the video. To do what the video talks about, I would expect that the second module would need to be written this way: