Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial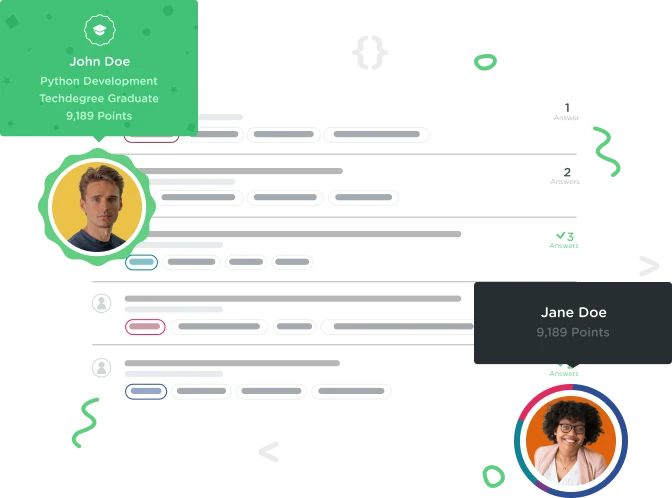

Charles Loder
17,541 PointsMongoose create() returning empty promise
So I'm working on console CRUD app using: Node, Mongo, Mongoose, commander.js, and inquirer.js.
In app.js, addItem()
is a function that returns Model.create({obj}), which according to the Mongoose docs, should return a promise. However, when I try to log the resp
I get undefined
. This was working fine before with a different model, but now not so much. I have a feeling create()
isn't working (I know it's not, because nothing is created), but I can't even figure out how to log the error. I've been stuck on this for a day or two and it doesn't seem like any one else has had this issue.
app.js
#!/usr/bin/env node
const mongoose = require('mongoose');
mongoose.Promise = Promise;
const program = require('commander');
const { prompt } = require('inquirer');
const {addItem, getItem, removeItem, makeArray} = require('./logic.js');
const {itemTypeQuestion, papyriQuestions, lampQuestions} = require('./questions');
const typeQuestions = (type) => {
if(type === 'papyrus') {
return prompt(papyriQuestions).then(resp => {resp.item_type = type; return resp})
} else if (type === 'lamp') {
return prompt(lampQuestions).then(resp => {resp.item_type = type; return resp})
}
}
program
.command('addItem')
.alias('a')
.description('Add an item')
.action( () => {
prompt(itemTypeQuestion)
.then(resp => typeQuestions(resp.item_type))
.then(answers => makeArray(answers, "more_ids", "other_ids", ", ") )
.then(resp => addItem(resp))
.then(resp => console.info(resp))
.then(mongoose.disconnect())
.catch(e => {
console.error(e);
mongoose.disconnect();
})
} )
logic.js
const mongoose = require('mongoose');
mongoose.Promise = Promise;
mongoose.connect('mongodb://localhost:27017/museum_catalog', { useNewUrlParser: true });
const {Papyrus, Lamp} = require('./schema.js');
/**
* @function [addItem]
* @returns {Promise} Promise
*/
const addItem = (item) => {
if (item.item_type === 'papyrus') {
return Papyrus.create(item)
} else if (item.item_type === 'lamp') {
return Lamp.create(item)
}
}
schema.js
const mongoose = require('mongoose');
// https://www.npmjs.com/package/mongoose-extend-schema
function extendSchema (Schema, definition, options) {
return new mongoose.Schema(
Object.assign({}, Schema.obj, definition),
options
);
}
// Define Item schema
const itemSchema = mongoose.Schema({
primary_id: {type: String, required: true, lowercase: true, unique: true, trim: true, index: true},
other_ids: [],
item_type: {type: String, required: true, lowercase: true, trim: true},
description: {type: String, lowercase: true, trim: true},
date_created: { type: Date, default: Date.now },
hidden: {type: Boolean, default: false},
height: Number,
width: Number
})
// Papyrus schema & model
const papyrusSchema = extendSchema(itemSchema, {
item_type: {type: String, default: 'papyrus', lowercase: true},
language: {type: String, required: true, lowercase: true, trim: true},
});
const Papyrus = mongoose.model('papyrus', papyrusSchema);
// Lamp schema & model
const lampSchema = extendSchema(itemSchema, {
item_type: {type: String, default: 'lamp', lowercase: true},
isInscription: Boolean,
language: {type: String, lowercase: true, trim: true},
})
const Lamp = mongoose.model('lamps', lampSchema);
module.exports = {Papyrus, Lamp};```
Also, any general feedback on other things is always welcomed