Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial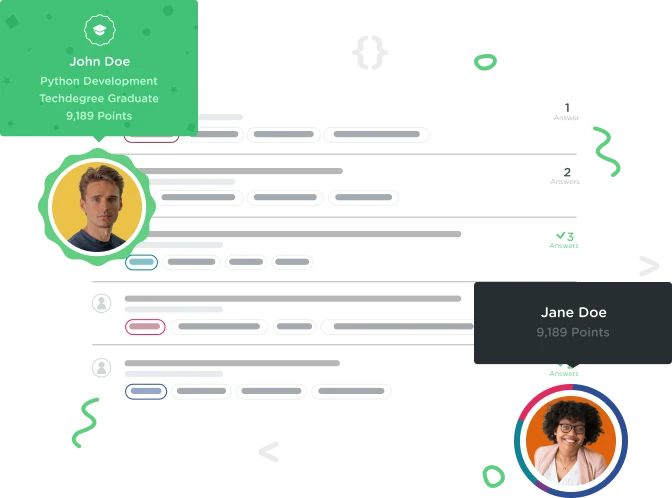
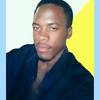
Dave Buda
5,157 PointsmOnItemClickListener
We're refactoring another list-based Activity to a grid. Some work has already been done. Now we want to show a button in the Action Bar when more than one player is selected in the GridView of this Activity. Start by adding an if statement in mOnItemClickListener that checks the count of checked items in mGridView.
import android.app.Activity;
import android.os.Bundle;
import android.view.ListView;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.GridView;
import android.widget.TextView;
public class InviteActivity extends Activity {
/*
* Some code has been omitted for brevity!
*/
public MenuItem mInviteMenuItem;
public GridView mGridView;
public TextView mEmptyTextView;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.player_grid);
mGridView = (GridView)findViewById(R.id.gridView);
mGridView.setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
mGridView.setOnItemClickListener(mOnItemClickListener);
}
public OnItemClickListener mOnItemClickListener = new OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// Start by adding code in here!
}
};
}
2 Answers

John Weland
42,478 PointsDave Buda, I hope this helps.
First we have to write an if statement
if ( /* SomeCondition */ ) {
// Do Something
}
now we need to add the condition we are checking
if (mGridView.getCheckedItemCount() > 0) {
// Do Something
}
what condition in the code above does is gets our mGridView(s) and then says give me count of any mGridView that is checked so long as the number of checked items is greater than 0
Now we have to add code that in the "Do Something" area so long as the count of the checked item (mGridView) is greater than 0.
if (mGridView.getCheckedItemCount() > 0 ) {
mInviteMenuItem.setVisibility(view.VISIBLE);
}
In the code above we take our item and set it visibility with mInviteMenuItem.setVisability(view.VISIBLE) where we simply pass view.VISIBLE like wise we need to add an else statement to set the view to invisible when the if statement conditions are not true.
if (mGridView.getCheckedItemCount() > 0 ) {
mInviteMenuItem.setVisibility(view.VISIBLE);
} else {
mInviteMenuItem.setVisibility(view.INVISIBLE);
}
we have to go back now above our OnItemClickListener method and define what our empty view is with
mEmptyTextView = (TextView) findViewById(android.R.id.empty);
and finally we set this view as the empty view for mGridView with
mGridView.setEmptyView(mEmptyTextView);

MUZ140223 Patience Mwale
4,647 Pointsthanks John u are a star