Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial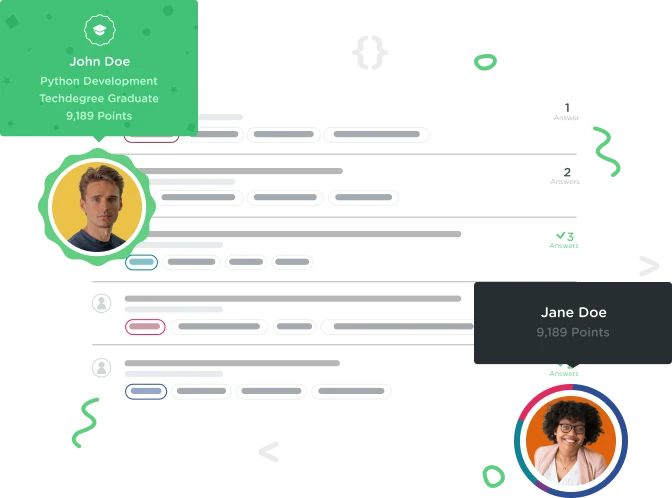
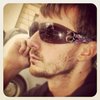
Adam Sackfield
Courses Plus Student 19,663 PointsMore clarity needed
At around 9mins into this video Jason Seifer updates the edit_spec.rb to the following
describe "Editing todo lists" do
def update_todo_list(options={})
options[:title] ||= "My todo list"
options[:description] ||= "This is my todo list."
todo_list = options[:todo_list]
visit "/todo_lists"
within "#todo_list_#{todo_list.id}" do
click_link "Edit"
end
fill_in "Title", with: options[:title]
fill_in "Description", with: options[:description]
click_button "Update Todo list"
end
it "updates a todo list successfully with correct information" do
todo_list = TodoList.create(title: "Groceries", description: "Grocery list.")
update_todo_list todo_list: todo_list, title: "New title", description: "New description"
todo_list.reload
expect(page).to have_content "Todo list was successfully updated"
expect(todo_list.title).to eq "New title"
expect(todo_list.description).to eq "New description"
end
end
But without much clarity as to what is happening, mainly the following line
update_todo_list todo_list: todo_list, title: "New title", description: "New description"
So obviously the "update_todo_list" part is calling the defined method, but then we have "todo_list:" what is this doing and also the "todo_list" which follows it is confusing as the "todo_list = " is set inside the aforementioned method and also in the it block for the test itself using the create method.
Could someone shed some light on what is happening with that line of code?
Thanks
1 Answer

Stone Preston
42,016 Pointsok so it looks like this line of code
update_todo_list todo_list: todo_list, title: "New title", description: "New description"
is calling the update_todo_list method that takes an options hash as an argument. it might help to rewrite the call with parenthesis for clarity, as well as the { } around the hash (since they are the only argument passed to the method you can omit them, but ill add them back in)
update_todo_list( { todo_list: todo_list, title: "New title", description: "New description" } )
so as you can see we are passing in the options hash and that hash contains several values. Looking at the definition here:
def update_todo_list(options={})
options[:title] ||= "My todo list"
options[:description] ||= "This is my todo list."
todo_list = options[:todo_list]
visit "/todo_lists"
within "#todo_list_#{todo_list.id}" do
click_link "Edit"
end
fill_in "Title", with: options[:title]
fill_in "Description", with: options[:description]
click_button "Update Todo list"
end
you can see in these lines:
options[:title] ||= "My todo list"
options[:description] ||= "This is my todo list."
we only set the title and description symbols if they are not present in the hash ( using ||= means that the value is only assigned if the variable is nil) , so in our case these lines dont run since we passed in a title and description in the hash.
Then the local todo_list variable is set using the todo_list we passed in the hash
todo_list = options[:todo_list]
then we simulate visiting the edit page and filling out hte form with the following lines
visit "/todo_lists"
within "#todo_list_#{todo_list.id}" do
click_link "Edit"
end
fill_in "Title", with: options[:title]
fill_in "Description", with: options[:description]
click_button "Update Todo list"
it uses values from the options hash we passed in to fill in the fields title and description, then we update the todo list
Adam Sackfield
Courses Plus Student 19,663 PointsAdam Sackfield
Courses Plus Student 19,663 PointsThanks for you detailed response, that clears it up somewhat, it's this here really "todo_list: todo_list" why the 2 todo_lists?
Stone Preston
42,016 PointsStone Preston
42,016 Points{ todo_list: todo_list,
the first part that statement
todo_list:
is a key for that options hash, the second part that follows is a variable called todo_list that you must have created earlier in the file (maybe with a let statement?). its a bit confusing that they are the same name, but the : after the first one means its a key (and a symbol, which is why you can omit the hash rocket when pairing it with a value). You could have also written{ :todo_list => todo_list,
Adam Sackfield
Courses Plus Student 19,663 PointsAdam Sackfield
Courses Plus Student 19,663 PointsPerfect! That has cleared it up for me. Just trying to understand everything that is happening. Rails is the main thing I want to learn and feel the video's just do stuff without explaining it.
Stone Preston
42,016 PointsStone Preston
42,016 Pointshonestly im pretty disappointed with treehouse's rails courses. I was hoping some of the newer courses would not rely on scaffolding so much. I started the todo list course last week, but I also felt they skip over a lot and there isnt much explanation provided. I decided to use the Rails Tutorial instead. Its very in depth, doesnt use scaffolding (except for the first demo project which is the intro), and so far has been extremely good.
Adam Sackfield
Courses Plus Student 19,663 PointsAdam Sackfield
Courses Plus Student 19,663 PointsYes, it's next on my list, was going to to the free text version rather than pay for more video;s. I also have This book but I got lost around half way in so thought would come here and try the Todo list app. I totally agree that scaffolds should be the last thing shown, first you should know how to do everything without them, then it would allow you to use them as needed in the future.
Stone Preston
42,016 PointsStone Preston
42,016 Pointsim using the free ebook version (which is fully updated for Rails 4 and Ruby 2.0). I really like it. I havent seen any of the videos.
Adam Sackfield
Courses Plus Student 19,663 PointsAdam Sackfield
Courses Plus Student 19,663 PointsStone Preston That ebook tutorial is miles better than the stuff on here, I am on chapter 7 and the stuff is actually sinking in, chapter 6 was hard, but I feel if I go through all 11 chapters several times then I will understand it enough to actually make something that works. Then move on to some Rails books. :)
Stone Preston
42,016 PointsStone Preston
42,016 Pointsyeah its a really good tutorial. I plan on doing it a few times as well.
Stone Preston
42,016 PointsStone Preston
42,016 Pointsit is rather long though. Im on chapter 10 and im having trouble staying focused. I just want it to be over.
Adam Sackfield
Courses Plus Student 19,663 PointsAdam Sackfield
Courses Plus Student 19,663 PointsIt's insanely long but that's what I like about it really. I have just finished Chapter 7, but I have been doing a chapter then having a break, sometimes overnight then resuming the next day took 2 days to get to this point. I see what your saying though Chapter 6/7 are very detailed and can be hard to remain focused, I find it good to take a step back if losing interest and stick on some dirty dubstep to relax lol. Or today been learning Spanish on duolingo just to keep my mind of Rails. RoR is the place I want to be though and the focus for a career, feel that's a long way off though. How long you been at it?
Stone Preston
42,016 PointsStone Preston
42,016 PointsI started the tutorial last week. Ive been putting about 2 hours/day into it. Almost done. I plan on going back again and doing a more detailed run through (less copying/pasting of the code more typing everything out) after this first time. Maybe even doing it a third time and taking some notes. It is really an amazing tutorial though, especially for a free one.
Adam Sackfield
Courses Plus Student 19,663 PointsAdam Sackfield
Courses Plus Student 19,663 PointsAh see I don't work so spend most of the day on it really, but no copying and pasting, I learn more by typing it out even the boring CSS which I already know. I intend going over it several times too, especially the user login/create stuff as that was intense. It truly is amazing for free, puts the Rails stuff on here to shame since these on here are aimed at beginners, not to openly diss TTH a the other content has been first class, learnt all my HTML, CSS, jQuery, WP here. Will try go back to the rails stuff here once I have a good understanding. Hopefully they will do some more Rails 4 ones without scaffolds. Defo a good idea to go through taking notes maybe even a mind map (flow chart) kind of notes to give you a sense of flow.
Stone Preston
42,016 PointsStone Preston
42,016 Pointsoh yeah every other course ive done here (iOS especially) has been amazing. and I dont really think that the Rails courses here are necessarily bad, its just this one really blows them out of the water in terms of content (testing especially. so many tests)