Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial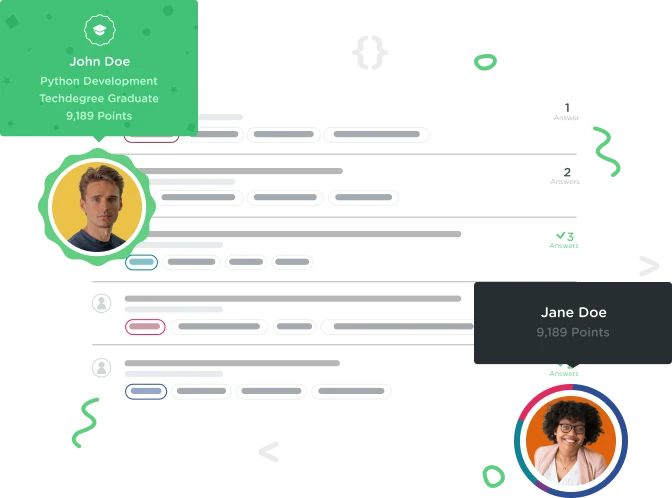
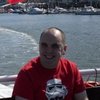
Sean Flanagan
33,235 PointsMore console trouble
Hi.
My console isn't working. Here's the code for Prompter.java:
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public boolean promptForGuess() {
Console console = System.console();
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
}
Hangman.java:
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public boolean promptForGuess() {
Console console = System.console();
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
}
Game.java:
public class Game {
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
public boolean applyGuess(char letter) {
boolean isHit = mAnswer.indexOf(letter) >= 0;
return isHit;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
}
I would be grateful where I've gone wrong please.
Thanks
Sean
4 Answers
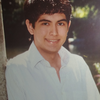
Jon Kussmann
Courses Plus Student 7,254 PointsHello,
Would you mind explaining more what you mean by "isn't working"?
One thing I've noticed in your Game.java file is:
public boolean applyGuess(char letter) {
boolean isHit = mAnswer.indexOf(letter) >= 0;
return isHit;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
You are returning your method before it ever gets to the if-else statement. You probably want to remove the first return statement and return only after the if-else statement has run.
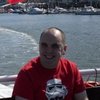
Sean Flanagan
33,235 PointsHi Jon.
OMG You're right; I've posted the same code twice! Sorry about my mistake.
Okay I'll try again, starting with Hangman:
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
prompter.displayProgress();
boolean isHit = prompter.promptForGuess();
if (isHit) {
System.out.println("We scored a hit!");
} else {
System.out.println("Missed!");
}
prompter.displayProgress();
}
}
Next, Prompter:
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public boolean promptForGuess() {
Console console = System.console();
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
public void displayProgress() {
System.out.printf("Try to solve: %s\n", mGame.getCurrentProgress());
}
}
Thanks for your support and I do beg your forbearance. Hopefully this is more helpful.
Sean :-)
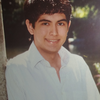
Jon Kussmann
Courses Plus Student 7,254 PointsHi,
When you are calling the "clear &&... etc" You are doing so "outside" of java-repl, correct?
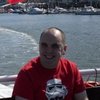
Sean Flanagan
33,235 PointsHi Jon.
Yes that's correct.
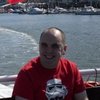
Sean Flanagan
33,235 PointsHi again Jon.
Just to let you know that the console is now working.
Your support will be remembered. Thank you :-)
Sean :-)
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsHi Jon. Thanks for trying to help.
I deleted the first return statement. The second is still where it was.
I tried to run the program again by typing "clear && javac Game.java && java Game" (I didn't type the double quotes :-) ). I got this message:
"Error: Main method not found in class Game, please define the main method as:
public static void main(String[] args)
or a JavaFX application class must extend javafx.application.Application"
Then I typed 'Game game = new Game("Treehouse");' and this message appeared in the console:
'Game game = new Game("Treehouse");
-bash: syntax error near unexpected token `(' '
Then "clear && javac Hangman.java && java Hangman" then I got this:
"ERROR: not a statement
clear && javac Hangman.java && java Hangman;
^
ERROR: ';' expected
clear && javac Hangman.java && java Hangman;
^
ERROR: not a statement
clear && javac Hangman.java && java Hangman;
^
ERROR: ';' expected
clear && javac Hangman.java && java Hangman;
^
ERROR: not a statement
clear && javac Hangman.java && java Hangman;
^
ERROR: ';' expected
clear && javac Hangman.java && java Hangman;
^
ERROR: not a statement
clear && javac Hangman.java && java Hangman;
^
ERROR: ';' expected
clear && javac Hangman.java && java Hangman;
^
ERROR: not a statement
clear && javac Hangman.java && java Hangman;
^
"
I don't know whether it's me or the console but I just can't make the program work.
Thanks for your feedback.
Sean
Jon Kussmann
Courses Plus Student 7,254 PointsJon Kussmann
Courses Plus Student 7,254 PointsHi Sean,
Your code for Hangman.java that you posted is the same as your Prompter class. Could you edit it to what you have there?
Also, when you type "clear && javac Game.java && java Game" I believe you should change each "Game" to "Hangman" since that is where your main (public static void main (String[] args)) method is.