Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial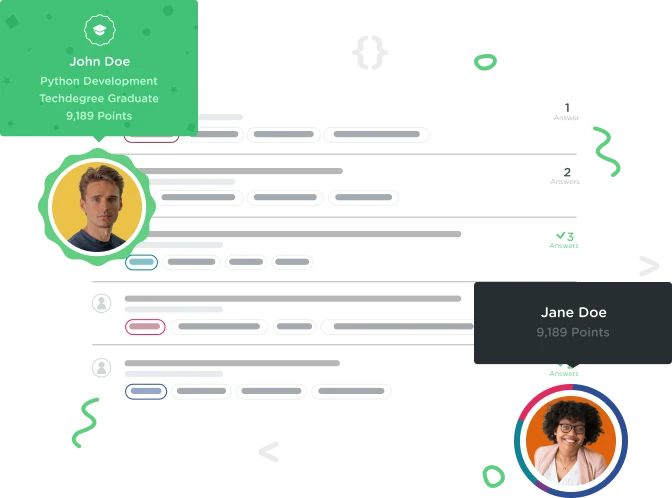
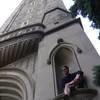
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMore efficient way of presenting the data
In the video, Dave uses a really inefficient and more difficult way to present the employees. I know he is doing it for learning purposes, but ES6 gives you a much better way that you will really appreciate when you are working with a lot of data and need to use conditionals. Check this out:
const employees = JSON.parse(request.responseText);
const employeeList = document.getElementById('employeeList');
let ul = '<ul class="bulleted">';
employees.forEach(employee => {
ul += `<li class=${employee.inoffice ? "in" : "out"}>${employee.name}</li>`;
});
ul += '</ul>';
employeeList.innerHTML = ul;
For one thing you can use a forEach
loop since you are going to iterate over every item in the data. Next, notice the ternary expression: `<li class=${employee.inoffice ? "in" : "out"}>${employee.name}</li>
;.
truthyValue ? doThis : doThat` is shorthand for "if truthyValue computes to true then do the first thing after the ? mark; else, do the thing after the colon. Using template literals you can dynamically set classes and other attributes simply by interpolating the ternary expression into the template literal. Then you can also interpolate the employee name.
If this stuff isn't familiar to you, take the Getting Started with ES2015 course. You'll be extremely glad you did because it makes working with strings and stuff waaay easier and more enjoyable! Course: https://teamtreehouse.com/library/getting-started-with-es2015-2
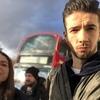
Jan Durcak
12,662 PointsThank you !
1 Answer
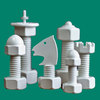
Steven Parker
231,275 PointsYou'll find that as you learn more techniques (not just ES2015), you will often be able to discover more compact and/or efficient ways to implement course exercises.
The fact that you're thinking of effective alternative strategies is evidence of your learning progress and grasp of the material. Good job!
Robert O'Toole
6,366 PointsRobert O'Toole
6,366 Pointsthanks so much. i remember learning this way in the JS 30 for 30 and knew it could be done this way. gonna just focus on ur approach ;)
treehouse is great but they def need to update some of these front end courses with new ES6 syntax.