Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial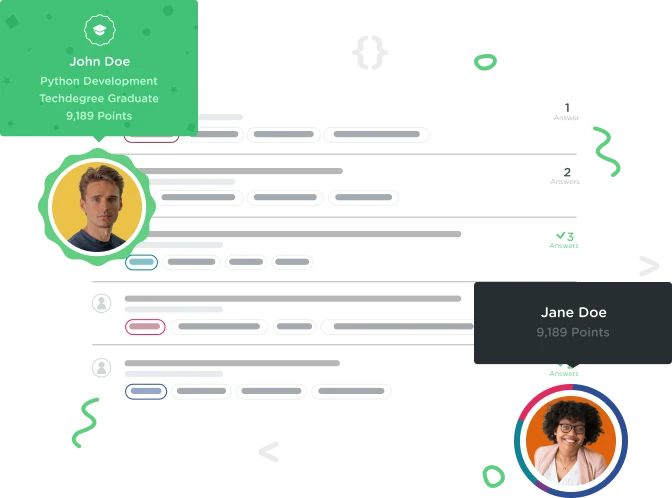

Ben Myhre
28,726 PointsMORE Nil value for response
I can hit the direct destination URL just fine and see the JSON data just fine. The original error I found was because the forecastURL was not unwrapped. It would not compile with a "value of optional type 'NSURL?' not unwrapped" I added the bang (forecastURL!) and and it would compile, but now when I run it, the response prints 'nil'. What is going on here?
import UIKit
class ViewController: UIViewController {
private let apiKey = "MY_KEY"
override func viewDidLoad() {
super.viewDidLoad()
let baseURL = NSURL(string: "https://api.forecast.io/forecast/\(apiKey)/")
let forecastURL = NSURL(string: "37.8267,-122.423", relativeToURL: baseURL)
let sharedSession = NSURLSession.sharedSession()
let downloadTask: NSURLSessionDownloadTask = sharedSession.downloadTaskWithURL(forecastURL!, completionHandler: { (location: NSURL!, response: NSURLResponse!, error: NSError!) -> Void in
println(response)
})
downloadTask.resume()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
2 Answers

Meek D
3,457 PointsIf you don't receive any data I think you should do an "if let" to try to unwrapped the data, so your program will not crash. Hope that helps....
And also why you don't have any data into this line of code ?
private let apiKey = "MY_KEY"

Art Hayes
7,251 PointsThis happened to me as well - I had to 'Clean' xcode to get the error to go away.
class ViewController: UIViewController {
private let apiKey = "xxxxxx"
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
let baseURL = NSURL(string: "https://api.forecast.io/forecast/\(apiKey)/")
let forecastURL = NSURL(string: "37.8267,-122.423", relativeToURL: baseURL)
// here's the actual network call
// here's our session object - and it's a singleton
let sharedSession = NSURLSession.sharedSession()
// create the task
let downloadTask: NSURLSessionDownloadTask = sharedSession.downloadTaskWithURL(forecastURL!, completionHandler: { (location: NSURL!, response: NSURLResponse!, error: NSError!) -> Void in
println(location, response)
})
downloadTask.resume()
}
}
I'll say this - after using Xcode heavily for the last few months - it is one buggy, temperamental beast.

Ben Myhre
28,726 PointsSo... I finally got back to this issue and after a full reboot, it seems to work. I had tried the old "restart XCode" last time to no avail. A full reboot and time was the solution I guess. sigh.
Ben Myhre
28,726 PointsBen Myhre
28,726 Pointsit is a private key. Sharing that on a public forum would be like punching myself in the face with hungry piranhas.