Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial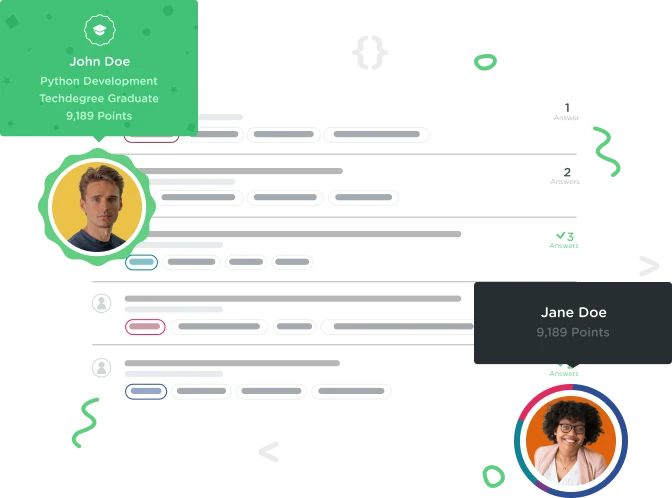

Hanna Han
4,105 PointsMore options in 'if' functions
Hi,
If I want to add more options in if/elif functions with "Done", "done", "DONE" how can I solve that in the best way? I think there must be users who write "done" in different way by combining lower/uppercase when they write it. For users I want to add other options.
Here is what I tried but it doesn't seem work:
def show_help(): print("What should we pick up at the store?") print(""" Enter "Done" to stop adding items. Enter "Help" for this help. """)
show_help()
while True:
new_item = input("> ")
if new_item == "done" or "Done" or "DONE":
break
elif new_item == "help" or "Help" or "HELP":
show_help()
continue
2 Answers

Anthony Crespo
Python Web Development Techdegree Student 12,973 PointsIt doesn't work cause the if statement is always true :
def show_help():
print("What should we pick up at the store?")
print(""" Enter "Done" to stop adding items. Enter "Help" for this help. """)
show_help()
while True:
new_item = input("> ")
# This always returns True cause you only compare new_item with "done"
# "Done" and "DONE" are not compared with new_item
# is like saying: if new_item == "done" or True or True
if new_item == "done" or "Done" or "DONE":
break
# same here
elif new_item == "help" or "Help" or "HELP":
show_help()
continue
You need to compare it with each possibility like this:
if new_item == "done" or new_item == "Done" or new_item == "DONE":
break
But that can be long if you want to cover each possibility like done, Done, dOne, doNe, etc...
What I personally do is that I use the string function .upper() on the user input when comparing. Upper return a copy of the string converted to uppercase. And then I only need to compare the user input with the word "DONE" in uppercase
Your code with the upper function will look like this:
def show_help():
print("What should we pick up at the store?")
print(""" Enter "Done" to stop adding items. Enter "Help" for this help. """)
show_help()
while True:
new_item = input("> ")
if new_item.upper() == "DONE":
break
elif new_item.upper() == "HELP":
show_help()
continue
Now your code work with all the possibilities of "done" and "help" and will be a lot more easier to maintain. Like if you want to change Done to Exit, you can do it without writing all the possibilities again. Hope this help.

Hanna Han
4,105 PointsThank you so much. It helped a lot :)

Anthony Crespo
Python Web Development Techdegree Student 12,973 PointsNo problem, happy i could help.