Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial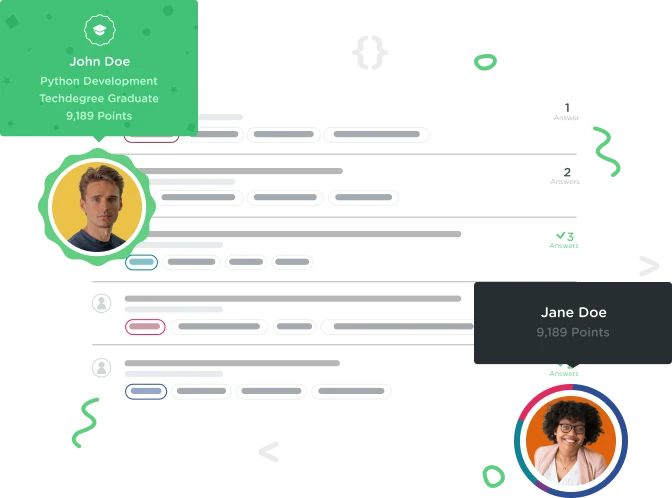

Gary Gibson
5,011 PointsMore Trouble With Wins And Losses Tally
My code runs fine in this hangman game, but I can't figure out how to get the wins and losses to show tally and show up.
import random
word_list = [
"banana",
"grocery",
"blockbuster",
"bridesmaid",
"stereotype",
"bad",
"cotton",
"pick",
"ridiculous",
"envoy",
"enjoy",
"braiding"
]
def play_again():
play_again = input("Play again? Y/n" )
if play_again == "Y":
game()
else:
quit()
def you_won():
print("You win!")
play_again()
def game():
wins = 0
losses = 0
while True:
secret_word = random.choice(word_list)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 5 and len(good_guesses) != len(secret_word):
for i in secret_word:
if i in good_guesses:
print(i, end='')
else:
print('-', end='')
print("")
player_guess = input(str("Please enter a letter. ")).lower()
if player_guess == "quit":
quit()
break
elif player_guess == "solve now":
guess_solution = input("Enter your word guess. ").lower()
if guess_solution == secret_word:
print(secret_word)
wins += 1
you_won()
else:
print("Sorry. That was incorrect!")
losses += 1
play_again()
elif len(player_guess) > 1:
print("You can only guess one letter at a time.")
continue
elif player_guess in bad_guesses or player_guess in good_guesses:
print("You already guessed that letter.")
continue
elif not player_guess.isalpha():
print("You can only guess letters, not numbers.")
continue
elif player_guess not in secret_word:
bad_guesses.append(player_guess)
print("{} was a bad guess. You have {} guesses left.".format(player_guess, 5-len(bad_guesses)))
else:
for i in secret_word:
if i == player_guess:
good_guesses.append(player_guess)
if len(good_guesses) == len(secret_word):
print(secret_word)
wins += 1
you_won()
else:
print("You didn't guess it. The word was '{}'.".format(secret_word))
losses += 1
play_again()
game()
2 Answers
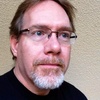
Chris Freeman
Treehouse Moderator 68,425 PointsThe issue is calling game()
from play_again()
. When called again, game()
resets the values of wins
and losses
to 0
.
Since all calls to play_again
originate from within game()
while
loop or from you_won()
(also called from within game
while
loop), let the function play_again()
finish naturally without calling game()
and control will return to within game
at the called point, the inner while
loop will complete, and control will fall to the outer while True
loop to start the next game without resetting the win loss values.
Finally, add a print statement as the last statement within the outer while
loop to print the totals before starting the next loop. Alternatively, you can also add the print after each update to either wins
or losses
.

Gary Gibson
5,011 PointsThank you, Chris.
Okay, I removed those two top functions calls and inserted a tally and printout after each block with the appropriate conclusion. Then I had to play around with the code so that it tallied and came to the proper conclusion no matter which way the player got it right (filling in all the correct letters, or "solve now").
import random
word_list = [
"banana",
"grocery",
"blockbuster",
"bridesmaid",
"stereotype",
"bad",
"cotton",
"pick",
"ridiculous",
"envoy",
"enjoy",
"braiding"
]
def game():
wins = 0
losses = 0
print("""
Welcome to hangman. Please enter one letter guess at a time. You have five chances to make an incorrect guess.
If you think you know the answer, you can enter 'solve now' to enter your guess...but if you make a wrong guess, you lose that game.
""")
while True:
secret_word = random.choice(word_list)
guess_solution = ''
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 5 and len(good_guesses) != len(secret_word):
for i in secret_word:
if i in good_guesses:
print(i, end='')
else:
print('-', end='')
print("")
player_guess = input(str("Please enter a letter. ")).lower()
if player_guess == "quit":
quit()
break
elif player_guess == "solve now":
guess_solution = input("Enter your word guess. ").lower()
if guess_solution == secret_word:
good_guesses = guess_solution
print("You got it! {}.".format(secret_word))
wins += 1
print("{} wins, {} losses.".format(wins, losses))
else:
guess_solution = "incorrect"
break
elif len(player_guess) > 1:
print("You can only guess one letter at a time.")
continue
elif player_guess in bad_guesses or player_guess in good_guesses:
print("You already guessed that letter.")
continue
elif not player_guess.isalpha():
print("You can only guess letters, not numbers.")
continue
elif player_guess not in secret_word:
bad_guesses.append(player_guess)
print("{} was a bad guess. You have {} guesses left.".format(player_guess, 5-len(bad_guesses)))
print("")
else:
if i in secret_word:
print("{} was a good guess.".format(player_guess))
print("")
for i in secret_word:
if i == player_guess:
good_guesses.append(player_guess)
if len(good_guesses) == len(secret_word):
print("You got it! {}.".format(secret_word))
wins += 1
print("{} wins, {} losses.".format(wins, losses))
print("")
if len(good_guesses) != len(secret_word) or guess_solution == "incorrect":
print("You didn't guess it. The word was '{}'.".format(secret_word))
losses += 1
print("{} wins, {} losses.".format(wins, losses))
print("")
game()
Everything seems to be working perfectly now. It tallies losses whether I lose from running out of turns or from guessing wrong with the "solve now" option.