Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial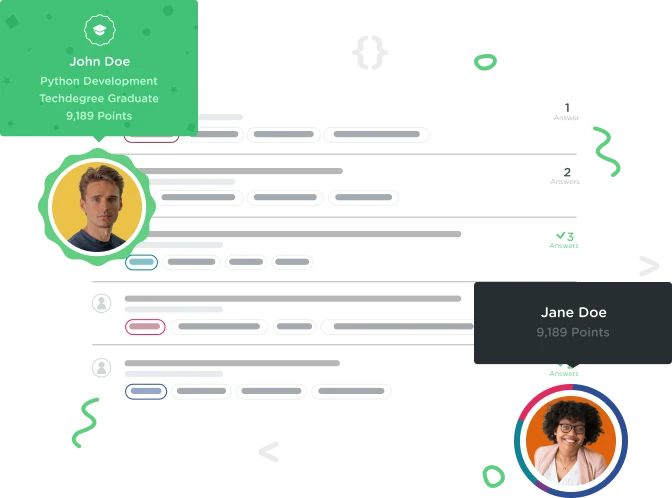
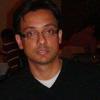
Mohammad Aslam
6,053 PointsMorse code and __str__
Hi there,
I don't quite understand what this challenge is asking for. Can someone please have a look at my code and advise me if I am on the right track and what to do next?
I also don't understand the str method. What is this particular method supposed to do? Why is it a special method? Can someone please explain?
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
list1 = []
for item in pattern:
if item == ".":
list1.append("dot")
if item == "_":
list1.append("dash")
return "-".join(list1)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
2 Answers

Wade Williams
24,476 PointsYou're definitely on the right track! You just need to add self to pattern in your for loop.
for item in self.pattern:
When you add a __str__ function to your class, you're telling python what it should do when str() is call on an instance of that class. If your class doesn't have a __str__ method, you'll get something like <__main__.S object at 0x0287F890> which is the instance's location in memory. This is undesirable, so we tell python to create a morse code string when str() is called on our class.
morse_code = S()
# without __str__ method in class
# <__main__.S object at 0x0287F890>
str(morse_code)
# with __str__ method in class
# "dot-dot-dot"
str(morse_code)
Dan Bader has a nice article on "dunder" methods:
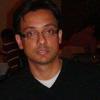
Mohammad Aslam
6,053 PointsThanks a lot!
So if you try running str() on an instance of the class, instead of performing a string function on the instance, it somehow produces the memory location. And by using str, you can change this output to something else you set arbitrarily.
It makes more sense, but I don't understand completely. Why would you want to call str() on an instance anyway? How does it help you?