Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial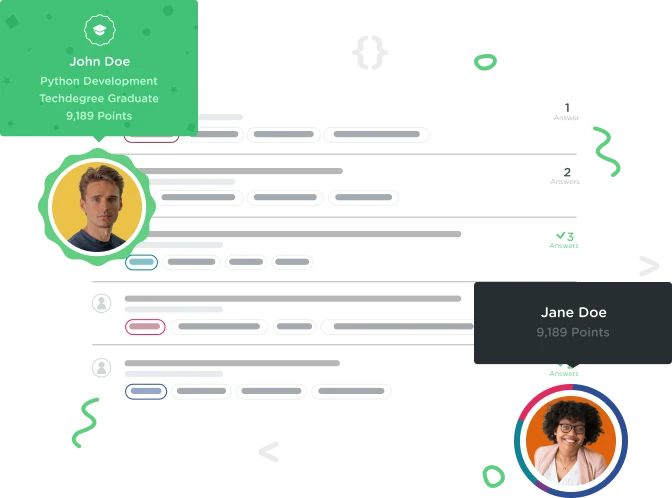

Shadow Skillz
3,020 Pointsmost courses
Please help!
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dic):
return len(dic)
def num_courses(dic):
course = 0
for teachers in dic:
course += len(dic[teachers])
return course
def courses(dic):
class1 = []
for classes in dic.values():
class1.extend(classes)
return class1
def most_courses(dic):
max_count = 0
teachers = " "
for teach, m_courses in dic.iteritems():
if teach > (max_count):
max_count = len(m_courses)
teachers = teach
return teachers
2 Answers
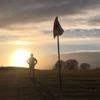
Stuart Wright
41,120 PointsI have made a few edits to your code to get it to pass:
1 - Changed .iteritems() to .items(). I think .iteritems() might be a Python 2 thing, but in Python 3, dictionary.items() is the way to go.
2 - Changed your if statement to compare the length of current teacher's courses to the max count. You were comparing the teacher's name to max count.
3 - Indented the 'teachers = teach' line. You only want this variable to change if that teacher is indeed the teacher with the current max count.
Full solution here:
def most_courses(dic):
max_count = 0
teachers = " "
for teach, m_courses in dic.items():
if len(m_courses) > (max_count):
max_count = len(m_courses)
teachers = teach
return teachers
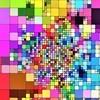
james south
Front End Web Development Techdegree Graduate 33,271 Pointsclose, you just need to tab the teachers = teach line over once to the right so it is under max count, not the if, and the challenge doesn't like iteritems so just just use items().

Shadow Skillz
3,020 Pointsthanks James
Michael Revy
3,882 PointsMichael Revy
3,882 PointsIf two or more teachers have same number of courses, the above gives you the last teacher found in the dict. I suppose if you wanted to return a list of teachers you could reset teachers whenever a new max is found, otherwise, if same max append name to a list.
Shadow Skillz
3,020 PointsShadow Skillz
3,020 Pointsthanks for your help Stuart I really appreciate it.