Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial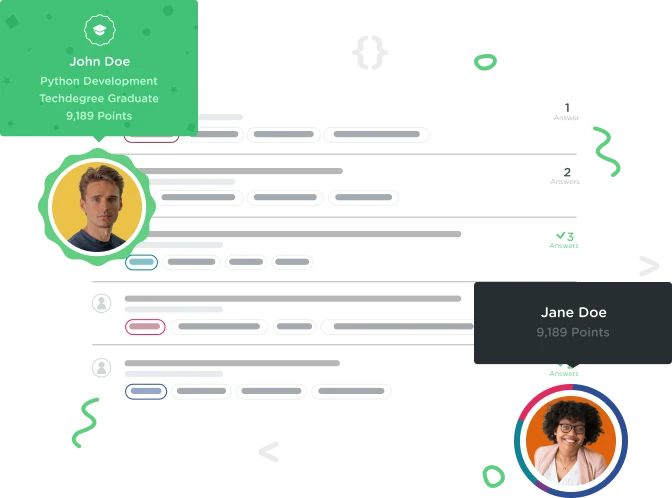

tyler bucasas
2,453 Pointsmovement.py
someone please help :)
# EXAMPLES:
# move((1, 1, 10), (-1, 0)) => (0, 1, 10)
# move((0, 1, 10), (-1, 0)) => (0, 1, 5)
# move((0, 9, 5), (0, 1)) => (0, 9, 0)
def move(player, direction):
x, y, hp = player
if direction == (1,0):
x += 1
elif x == 9:
hp -= 5
if direction == (-1,0):
x -= 1
elif x == 0:
hp -+ 5
if direction == (0,1):
y +=1
elif y == 9:
hp -+ 5
if direction == (0,-1):
y -= 1
elif y == 0:
hp -= 5
return x, y, hp
2 Answers
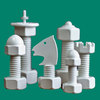
Steven Parker
231,269 PointsA few hints:
- an "elif" will always line up (start in the same column) as the corresponding "if"
- on the other hand, a nested "if" will be indented (but just spelled "if")
- the symbols "-+" written together are not a valid Python operator
- 0 and 9 are both allowed values within the limits
- if the movement goes outside the limits, the original x and y should be returned unchanged
- movement might occur in both directions at the same time
- movement might be for more than one unit in one or both directions ā”
ā” = I'm not sure if the validator tests that last one, but the instructions don't eliminate the possibility.

tyler bucasas
2,453 Pointsdef move(player, direction):
x, y, hp = player
if direction == (1,0) and x <= 9:
x += 1
else:
hp -= 5
if direction == (-1,0) and x >= 0:
x -= 1
else:
hp -= 5
if direction == (0,1) and y <= 9:
y += 1
else:
hp -= 5
if direction == (0,-1) and y >= 0:
y -= 1
else:
hp -= 5
return x, y, hp
Steven Parker pls help
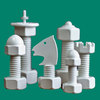
Steven Parker
231,269 PointsYou still have to handle the possibility that the movement may be in both dimensions at the same time. And you might need to account for more than one unit of motion as well.
You might want to split up "direction" and check each dimension separately.