Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial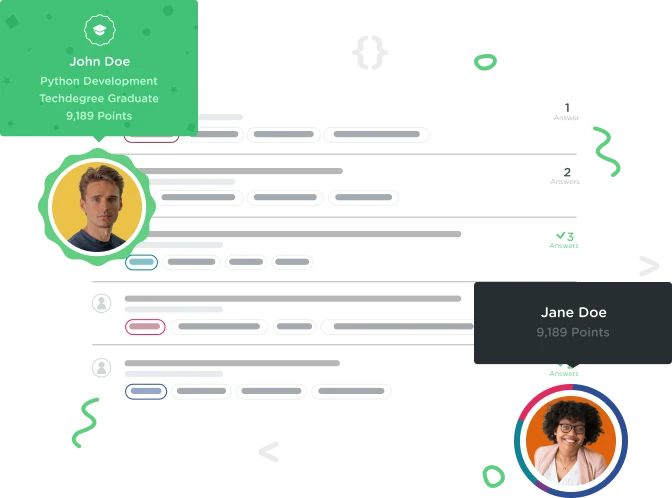
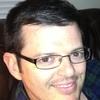
Brian Hudson
14,785 Pointsmoves.remove() vs. moves = moves.remove()
Kenneth removed the pertinent items from the moves list "in place":
def get_moves(player):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
x, y = player
if y == 0:
moves.remove("UP")
if y == 4:
moves.remove("DOWN")
if x == 0:
moves.remove("LEFT")
if x == 4:
moves.remove("RIGHT")
return moves
but I originally had the get_moves function written as:
def get_moves(player):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
x, y = player
if y == 0:
moves = moves.remove("UP")
if y == 4:
moves = moves.remove("DOWN")
if x == 0:
moves = moves.remove("LEFT")
if x == 4:
moves = moves.remove("RIGHT")
return moves
where I set moves = moves.remove()
I would have thought these basically are saying the same thing, but when I originally tried running the code I got a "TypeError: can only join an interable", or something like that. I'm confused...what's the difference between the two?
2 Answers

Ryan S
27,276 PointsHi Brian,
The difference depends on whether or not a method returns a value. In the case of .remove()
, it does not return a value, it only modifies the iterable to which it is applied. The same is true for .append()
.
So in your version, the "moves" variable will be of type "NoneType" because nothing was returned in the method. Your error is not likely a encountered inside the "get_moves" function, because what you are attempting to do will not produce an error, but its probably raised outside where it is being called, since "moves" will have a value of None.
The .pop()
method, on the other hand, does return a value so you can pull out an item and store it in a variable. However, this wouldn't be appropriate for the logic of the function, since you want to utilize the remaining moves in the list, not the one you removed.
So even if .remove()
returned a value, it would essentially invert the logic of the game by grabbing the only move that you shouldn't be able to use, rather than leaving behind the ones you should.
Hope this clears things up.
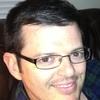
Brian Hudson
14,785 PointsNot only did it clear things up, you also turned on a lightbulb regarding the .pop() method. Thanks for the great reply!