Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial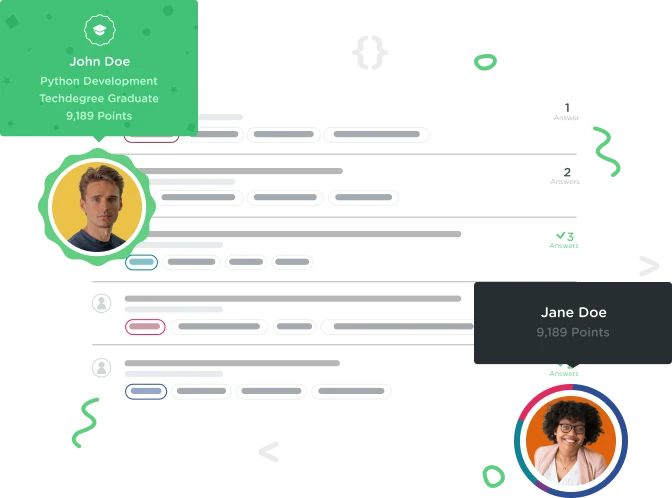
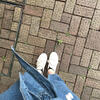
Tani Huang
6,952 Pointsmovie.js line1 - It show "Maximum call stack size exceeded"..what does it mean ?
<ol id="playlist"></ol>
<button id="play">Play</button>
<button id="next">Next</button>
<button id="stop">Stop</button>
<script src="playlist.js"></script>
<script src="media.js"></script>
<script src="movie.js"></script>
<script src="song.js"></script>
<script src="app.js"></script>
song.js
function Song (title,artist,duration) {
Media.call(this,title,duration); //string array
this.artist = artist;
};
Song.prototype = Object.create(Media.prototype);
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying) {
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - '
htmlString += this.artist;
htmlString += '<span class="duration">'
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
movie.js
function Movie (title,year,duration) {
Movie.call(this,title,duration); //string array
this.year = year;
};
Movie.prototype = Object.create(Media.prototype);
Movie.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying) {
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' ( ';
htmlString += this.year;
htmlString += ' ) ';
htmlString += '<span class="duration">'
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
media.js
function Media (title,duration) {
this.title = title;
this.duration = duration;
this.isPlaying = false;
};
Media.prototype.play = function() {
this.isPlaying = true;
};
Media.prototype.stop = function() {
this.isPlaying = false;
};
app.js
var playlist = new Playlist();
var song1 = new Song ("title1","artist1","duration1");
var song2 = new Song ("title2","artist2","duration2");
var movie1 = new Movie ("title1",2011,"duration1");
var movie2 = new Movie ("title2",2012,"duration2");
playlist.add(song1);
playlist.add(song2);
playlist.add(movie1);
playlist.add(movie2);
var playlistElement = document.getElementById("playlist");
playlist.renderInElement(playlistElement);
var playButton = document.getElementById("play");
playButton.onclick = function() {
playlist.play();
playlist.renderInElement(playlistElement);
}
var playButton = document.getElementById("next");
playButton.onclick = function() {
playlist.next();
playlist.renderInElement(playlistElement);
}
var playButton = document.getElementById("stop");
playButton.onclick = function() {
playlist.stop();
playlist.renderInElement(playlistElement);
}
1 Answer

Jeff Wilton
16,646 PointsBasically that error is indicating that a particular function is being called over and over again without end, until the call stack limit is exceeded. In this case, you have a (accidental) recursive endless loop caused by the Movie function calling itself without end.
Here is the error:
function Movie (title,year,duration) {
Movie.call(this,title,duration); // Oops, this should be Media.call
this.year = year;
};
It can really be difficult to catch those little details, and JS error messages are notoriously vague, but in this case the error message indicated the problem was with the Movie function. and since it's such a small function it was an easy problem to figure out. Hope this helps!