Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial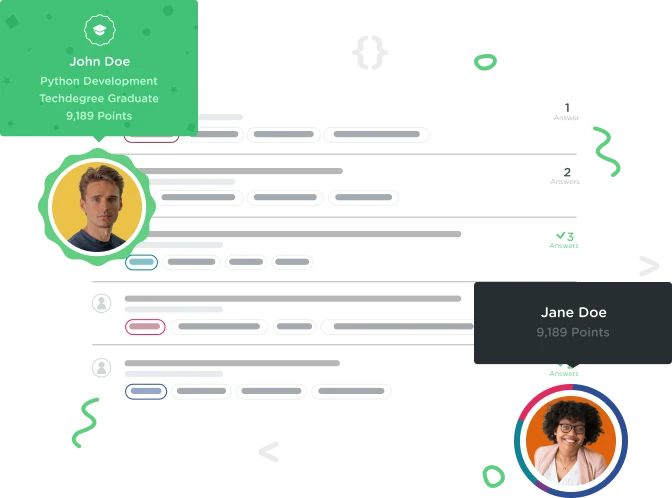

Steven Wheeler
2,330 PointsMoving items in a list?
def show_list():
count = 1
for item in shopping_list:
print("{}: {}".format(count, item))
count += 1
def move_item():
show_list()
print("Give me the number of the item you want to move")
userNumber = input(">>> ")
print("Now give me the number where you want this item to be put")
destNumber = input(">>> ")
idx = int(userNumber) - 1
item = shopping_list.pop(idx)
finalDestinationNumber = int(destNumber) - 1
shopping_list.insert(finalDestinationNumber, item)
print("Item moved!")
show_list()
def add_list():
while True:
newItem = input(">>> What would you like to add to the list? ")
if (newItem == "DONE"):
show_list()
break
elif (newItem == "SHOW"):
show_list()
continue
elif (newItem == "CLEAR"):
del shopping_list[:]
print("Shopping list cleared")
continue
elif (newItem == "MOVE"):
move_item()
continue
else:
shopping_list.append(newItem)
continue
show_help()
add_list()
Hey there, I'm really not sure why my move_item() function isn't working as expected. I'm sure its something quite simple but... I just don't get why it's doing what it's doing. I've been trying for about 30 minutes and my head hurts haha. The problem has been making the number they give into an integer and minus 1 from it so that it will align with the item's actual index. There must be a simple gap in my logic but I can't see what it is right now.
I've only pasted the relevant code.
EDIT: I've updated the code but it still has the same problem :)
2 Answers
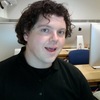
Nathan Smutz
9,492 PointsFirst problem: Both inputs assign to the same variable.
You use userNumber for both the item and where you want it to go. Right now idx and newNumber both will equal the "where you want this" number.
A quick fix would be to move `idx = int(userNumber) - 1 so that it's before the second input ststement.
Second problem: Your insert index is a moving target
When you pop the item, you change (lower) the index of every item after it.
Moving an item higher in the list:
Once you've popped it, everything higher has shifted down. When you went to insert it at the index you originally intended, that original index points at one item to the right.
Loking at a list like this:
0, 1, 2, 3
[a, b, c, d]
If you try to move a to after b: Your program would pop from 0 and insert at 2
0, 1, 2, 3
[a, b, c, d]
pop(0)
0, 1, 2
[b, c, d]
insert(2, a)
0, 1, 2, 3
[b, c, a, d]
So we meant to insert after b; but, since the items changed indices, we inserted after c.
So, you'll want to check whether you're inserting after the original item and, in that case, subtract 1 from the index you use when you insert.
if(idx < newNumber):
shopping_list.insert(newNumber-1 , item)
else:
shopping_list.insert(newNumber, item)
It seems like python should have a built in function for this; but a search hasn't turned anything up yet.
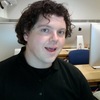
Nathan Smutz
9,492 PointsI saw your edit. What is the behavior that you're seeing? Are items not moving at all still? If it's just going to the wrong place (or erroring when moving to the end): I see you're not yet compensating for changed indices when moving higher.
Steven Wheeler
2,330 PointsSteven Wheeler
2,330 PointsThanks for the help, I'm not sure why I changed both inputs to assign the same variable (it hadn't always been like that).
It does seem to be very awkward with lists. I am guessing in reality you would maybe use a dictionary or something else to do this more efficiently.