Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial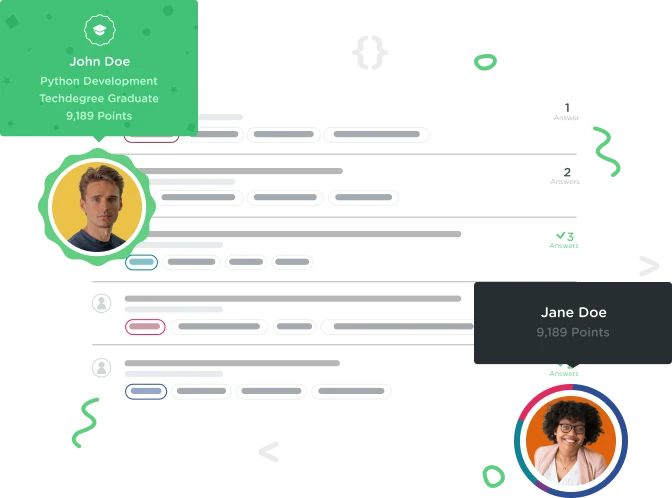
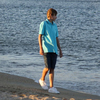
Tom Coomer
1,331 PointsMoving label with button
I would like to have a label move up (with a sliding animation) by 20 pixels when a button is pressed. How would I do this?
1 Answer

Mike Bronner
16,395 PointsThis is what I use to move the view up when showing the keyboard (put it in you buttonPressed method):
float viewWidth = self.view.frame.size.width;
float viewHeight = self.view.frame.size.height;
NSDictionary *keyboardInfo = [notification userInfo];
CGSize keyboardSize = [[keyboardInfo objectForKey:UIKeyboardFrameBeginUserInfoKey] CGRectValue].size;
float keyboardHeight = keyboardSize.height;
if (UIDeviceOrientationIsLandscape([[UIDevice currentDevice] orientation])) {
keyboardHeight = keyboardSize.width;
// Also swap the view width and height.
float temp = viewWidth;
viewWidth = viewHeight;
viewHeight = temp;
}
CGRect viewableAreaFrame = CGRectMake(0.0, 0.0, viewWidth, viewHeight - keyboardHeight);
CGRect txtDemoFrame = self.resultText.frame;
if (!CGRectContainsRect(viewableAreaFrame, txtDemoFrame)) {
// We need to calculate the new Y offset point.
//==>this is where you want to move it by 20, add the height of any toolbars at the bottom if needed
float scrollPointY = viewHeight + 20;
//float scrollPointY = viewHeight + self.ScanBar.frame.size.height - keyboardHeight;
// Don't forget that the scroller should go to its original position
// so store the current Y point of the content offset.
self.originalScrollerOffsetY = self.scroller.contentOffset.y;
// Finally, scroll.
[self.scroller setContentOffset:CGPointMake(0.0, scrollPointY) animated:YES];
}
For this to work, you will have to put all your objects that are currently in your view inside a scrollview. You can find the complete tutorial here: http://gabriel-tips.blogspot.com/2012/07/how-to-scroll-obscured-textfield-above.html
You won't need to implement the gesture recognizers, or the keyboard methods, just the UIScrollView and its delegate protocol. Also, don't forget to add an outlet for the UIScrollView.
Hope this helps a bit. :)
Tom Coomer
1,331 PointsTom Coomer
1,331 PointsOkay. I'll try it. Thanks