Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial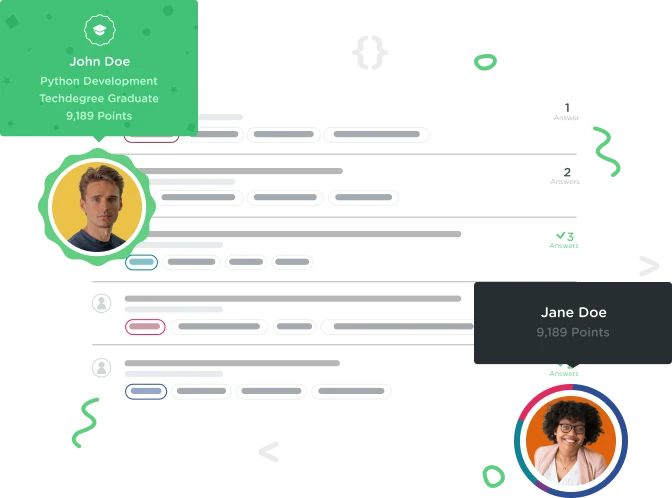

Connor Nesbitt
4,824 PointsMoving Work from the Main Thread to an AsyncTask code challenge help
I'm stuck on part one of this code challenge. The question is: Create a private class inside MainListActivity named 'CustomAsyncTask' and have it extend AsyncTask. Don't put anything in it yet, but AsyncTask requires 3 generic types: use 'Object', 'Void', and 'String'. Hint: The format will be AsyncTask<type1, type2, type3>. Here is my code: private class CustomAsyncTask extends AsyncTask<Object, Void, String>{} it keeps giving me a Complication error and I have no idea why. Can someone help me please?
4 Answers

Ernest Grzybowski
Treehouse Project ReviewerPlease try to post as much of your code as it may be easier to find where your problem may be. That's the only way we may be able to see a bracket missing. Nonetheless, the first thing I notice from reading the question is that there are two parts to the solution. It seems that you only did the first half.
Let's start with the code they give you:
public class MainListActivity extends ListActivity {
public static final String URL = "http://www.teamtreehouse.com";
public static final String TAG = MainListActivity.class.getSimpleName();
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
try {
URL treehouseUrl = new URL(URL);
HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection();
connection.connect();
int responseCode = connection.getResponseCode();
}
catch (MalformedURLException e) {
Log.e(TAG, "MalformedURLException caught!", e);
}
catch (IOException e) {
Log.e(TAG, "IOException caught!", e);
}
}
}
They ask "Create a private class inside MainListActivity named 'CustomAsyncTask' and have it extend AsyncTask." Let's do this below
public class MainListActivity extends ListActivity {
public static final String URL = "http://www.teamtreehouse.com";
public static final String TAG = MainListActivity.class.getSimpleName();
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
try {
URL treehouseUrl = new URL(URL);
HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection();
connection.connect();
int responseCode = connection.getResponseCode();
}
catch (MalformedURLException e) {
Log.e(TAG, "MalformedURLException caught!", e);
}
catch (IOException e) {
Log.e(TAG, "IOException caught!", e);
}
}
private class CustomAsyncTask extends AsyncTask {
}
}
Next they say "Don't put anything in it yet, but AsyncTask requires 3 generic types: use 'Object', 'Void', and 'String'."
public class MainListActivity extends ListActivity {
public static final String URL = "http://www.teamtreehouse.com";
public static final String TAG = MainListActivity.class.getSimpleName();
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
try {
URL treehouseUrl = new URL(URL);
HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection();
connection.connect();
int responseCode = connection.getResponseCode();
}
catch (MalformedURLException e) {
Log.e(TAG, "MalformedURLException caught!", e);
}
catch (IOException e) {
Log.e(TAG, "IOException caught!", e);
}
}
private class CustomAsyncTask extends AsyncTask<Object, Void, String> {
}
}
That should pass the first challenge question. Hope the breakdown helps!

Connor Nesbitt
4,824 PointsI forgot the <Object, Void, String> code in between the AsyncTask and the open curly brace. but it still gives me the error. private class CustomAsyncTask extends AsyncTask<Object, Void, String> {}

Connor Nesbitt
4,824 PointsYup, that was my problem. Thank you so much for helping me through this stressful time!

Connor Nesbitt
4,824 PointsYup, that was my problem. Thank you so much for helping me through this stressful time!
Connor Nesbitt
4,824 PointsConnor Nesbitt
4,824 PointsThanks for your help! But it is still saying that the answer is wrong for some reason. It keeps on giving me this every time I put the code in:Compilation Error! Verify that you have the generic parameters '<Object, Void, String>' between 'extends AsyncTask' and the opening curly brace, and check the rest of your syntax. And I put in the code exactly how you've done it.
Ernest Grzybowski
Treehouse Project ReviewerErnest Grzybowski
Treehouse Project ReviewerMake sure the imports are there!
Connor Nesbitt
4,824 PointsConnor Nesbitt
4,824 PointsThe imports are there and it is still giving me an error message. :/
Ernest Grzybowski
Treehouse Project ReviewerErnest Grzybowski
Treehouse Project ReviewerPlease post your entire code, it's difficult to tell why it's not going though.
This works for me without a hitch.
Connor Nesbitt
4,824 PointsConnor Nesbitt
4,824 PointsI figured it out. I had the code in the wrong spot....Thank you much for your help! Though I am having some issues with the second part of the code challenge. Here is my code:
(For some reason it does not post the second part of the AsyncTask code onto the reply. It is there though)
Ernest Grzybowski
Treehouse Project ReviewerErnest Grzybowski
Treehouse Project ReviewerConnor Nesbitt you are really close, but you first need to under stand that a method can either return something or it can return nothing. The challenge states:
You wrote:
"";
but that does not return a string. That actually won't even compile. You must use
return
followed by what you want to return.Examples:
With all of that laid out in front of you, you should be able to make it past the second challenge!
Jeremy Miller
2,455 PointsJeremy Miller
2,455 PointsI must be having a bad moment im stuck this keeps giving me this error.......
Bummer! Compilation Error! Verify that the 'doInBackground()' method starts with 'protected String' and actually returns a String inside the curly braces, and check the rest of your syntax
private class CustomAsyncTask extends AsyncTask<Object,Void,String>{
}