Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial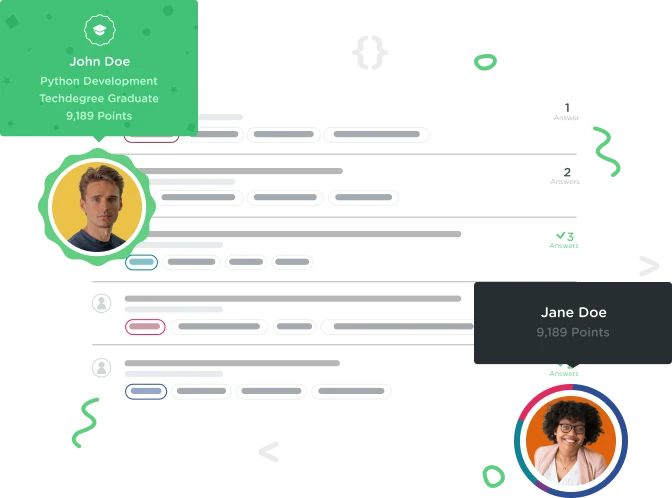

daniel steinberg
14,651 PointsMRO: copy, list
when i call mro in the following code it lists: (<class 'filledlist.FilledList'>, <class 'list'>, <class 'object'>)
why does it not contain copy instead of list? how does it default to list:
thx!
import copy
class FilledList(list):
def __init__(self, count, value, *args, **kwargs):
super().__init__()
for _ in range(count):
self.append(copy.copy(value))
import filledlist
from filledlist import FilledList
fl = filledlist.FilledList(9,2)
print(len(fl))
print(fl)
print(FilledList.__mro__)
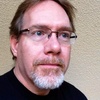
Chris Freeman
Treehouse Moderator 68,441 PointsYes, in a class definition, all of the parameters must be a class. In this case, βlistβ refers to the class list

daniel steinberg
14,651 Pointsok..I think I figures it out. here is the complete code:
import random
class Die:
def __init__(self, sides=2, value=0):
if not sides >= 2:
raise ValueError("Must have at least 2 sides")
if not isinstance(sides, int):
raise ValueError("Sides must be a whole number")
self.value = value or random.randint(1, sides)
def __int__(self):
return self.value
def __eq__(self, other):
return int(self) == other
def __ne__(self, other):
return int(self) != other
def __gt__(self, other):
return int(self) > other
def __lt__(self, other):
return int(self) < other
def __ge__(self, other):
return int(self) > other or int(self) == other
def __le__(self, other):
return int(self) < other or int(self) == other
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return int(self) + other
class D6(Die):
def __init__(self, value=0):
super().__init__(sides=6, value=value)
Note this code does not include below which is used in previous examples. If you add that line you get values instead of memory.
def __repr__(self):
return str(self.value)
Two questions
based on your previous answer, since Hand passes the object list (which has
__str__
), why do you need the__repr__
in Die(D6 is an extension of Die) ? This is confusing for meAre
__str__
and__repr__
more or less the same?
thx again for your help
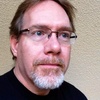
Chris Freeman
Treehouse Moderator 68,441 PointsWhen hand
is typed in the REPL, the __repr__
method is called, if referenced by print
or the format
method then the __str__
method is called. Some times the __repl__
method is defined to call the __str__
method.
>>> class obj:
... def __str__(self):
... return 'str string'
... def __repr__(self):
... return 'repr string'
...
>>> o = obj()
>>> o
repr string
>>> print(o)
str string
If no __str__
method is explicitly defined, the default object.__str__
method will call the __repr__
method.
More info in the docs
1 Answer
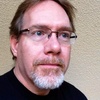
Chris Freeman
Treehouse Moderator 68,441 PointsGood question. The use of a built-in or imported function does not make it part of the object.
The methods and attributes of list
become part of FilledList
along with the methods and attributes of object
that are part all objects.
If copy
were part of FilledList
you would be able to call it using self.copy
. Note that there is a list.copy
method but this returns a shallow copy of the whole list and does not make a copy of some other argument.
Post back if you need more help. Good luck!!

daniel steinberg
14,651 PointsChris one more question if you dont mind...when I print fl in the following code I actually get the list:
import copy
class FilledList(list):
def __init__(self, count, value, *args, **kwargs):
super().__init__()
for _ in range(count):
self.append(copy.copy(value))
fl = FilledList(9,2)
print(fl)
[2, 2, 2, 2, 2, 2, 2, 2, 2]
Other times when I print an instance of an object I get a memory address, for ex:
<thieves.Thief object at 0x00D60990>
why do I not get a memory address in the example above
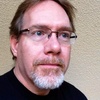
Chris Freeman
Treehouse Moderator 68,441 PointsThe instance fl
inherits the __str__
method from list
that is used by print
to display the items within the filled list. The class Thief
does not define or inherit a __str__
method.
Try:
thief_obj.__str__
where thief_obj
is the Thief
instance, it should return an undefined attribute error.

daniel steinberg
14,651 Pointsty!

daniel steinberg
14,651 Pointsty again

daniel steinberg
14,651 PointsChris can I bother you with a related question please..
When Kenneth runs the following he gets a list of object memory info
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
import dice, hands
hand=hands.Hand(size=5, die_class=dice.D6)
hand
[<dice.object at .......>, <.........]
When I run it i get values:
import dice, hands
hand=hands.Hand(size=5, die_class=dice.D6)
hand
[1, 1, 3, 5, 6]
Question...based on your previous answer...since list is being passed, and list has a __str__
, why does Kenneth get memory stuff?
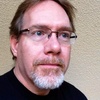
Chris Freeman
Treehouse Moderator 68,441 PointsThe list.__str__
method is what gives you the brackets and comma separated items. It would be the lack of a __str__
method in D6
that causes the βdice.object...β.
First to check: does your hand
contain a list of D6
instances or a list of int
instances. Try also running:
[type(x) for x in hand]
If you get int
s then int.__str__
is running.
Otherwise, Iβd have to see the D6
code to know more.

daniel steinberg
14,651 PointsChris may I bother you with one more question please. I am trying to understand the difference between appending a value and appending a copy of a value. in the Subclassing built in section Kenneth uses copy.copy because he says if a mutable was passed in and later changed all instances of the mutable would also change because they all reference the same thing. In the code below I am appending multiple versions of the same mutable(I commented out Kenneth's copy.copy), then I try changing the original version as well as one "copy". Neither causes other "copies" to change. Can you explain please? import copy
code:
class FilledList(list):
def __init__(self, count, value, *args, **kwargs):
self.value=value
super().__init__()
for _ in range(count):
# self.append(copy.copy(value))
self.append(value)
fl2 = FilledList(4,[123])
print(len(fl2))
print(fl2)
fl2.value=[567]
print(fl2.value)
print(fl2)
fl2[0] = [345]
print(fl2[0])
print(fl2)
output:
4
[[123], [123], [123], [123]]
[567]
[[123], [123], [123], [123]]
[345]
[[345], [123], [123], [123]]
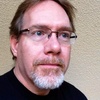
Chris Freeman
Treehouse Moderator 68,441 PointsWithout using copy
every item in the list points to the same object as shown by object id
. Replacing the whole item will, of course, not affect the other items. However, changing an item within the mutable object does affect them all. See adjusted code:
import copy
class FilledList(list):
def __init__(self, count, value, *args, **kwargs):
self.value=value
super().__init__()
for _ in range(count):
# self.append(copy.copy(value))
self.append(value)
class FilledListCopy(list):
def __init__(self, count, value, *args, **kwargs):
self.value=value
super().__init__()
for _ in range(count):
self.append(copy.copy(value))
fl2 = FilledList(4,[123])
print(len(fl2))
print(fl2)
print([id(x) for x in fl2])
fl2[0][0] = 'a'
print(fl2[0])
print(fl2)
print([id(x) for x in fl2])
fl2 = FilledListCopy(4,[123])
print(len(fl2))
print(fl2)
print([id(x) for x in fl2])
fl2[0][0] = 'a'
print(fl2[0])
print(fl2)
print([id(x) for x in fl2])
Output
4
[[123], [123], [123], [123]]
[4722088904, 4722088904, 4722088904, 4722088904]
[βaβ]
[[βaβ], [βaβ], [βaβ], [βaβ]]
[4722088904, 4722088904, 4722088904, 4722088904]
4
[[123], [123], [123], [123]]
[4662704392, 4662704904, 4662705224, 4662714440]
[βaβ]
[[βaβ], [123], [123], [123]]
[4662704392, 4662704904, 4662705224, 4662714440]
daniel steinberg
14,651 Pointsdaniel steinberg
14,651 Pointsok, Chris so "list" that is being passed to the Class the List object?