Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial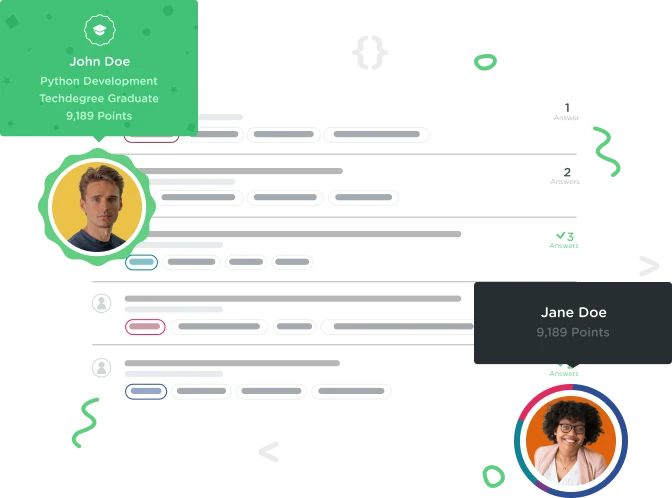

james tarala
1,370 PointsMultiple array elements not passing.
I can't seem to figure out why the two elements in the array aren't passing; the email address and the name. I presume it's because of the colon. I am sure I am missing something simple. Any help will be appreciated, so thanks in advance!
<?php
//edit this array
$contacts [] = array(
'name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com'
);
$contacts [] = array(
'name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com'
);
$contacts [] = array(
'name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com'
);
$contacts [] = array(
'name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com'
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>$contacts[0]['name'] : $contacts[0]['email']</li>\n";
echo "<li>$contacts[1]['name'] : $contacts[1]['email']</li>\n";
echo "<li>$contacts[2]['name'] : $contacts[2]['email']</li>\n";
echo "<li>$contacts[3]['name'] : $contacts[3]['email']</li>\n";
echo "</ul>\n";
?>

andren
28,558 PointsThat answer shows how the challenge can be solved with string concatenation (using . to combine strings) but the challenge can be solved with string interpolation (including variables in double quoted strings) like you tried as well, which I give an example of in my answer below.
1 Answer

andren
28,558 PointsActually this is a case of a somewhat common gotcha that PHP has regarding string interpolation in double quoted strings.
When PHP encounters a $ symbol in a double quoted string it has to guess where the variable actually stops. Normally it manages to do so fine on its own, but when you have a multidimensional array PHP will stop once it gets to the first pair of square brackets and then interpret the next set of brackets as pure text that is not related to the variable it should interpret, which is incorrect.
In order to tell PHP that you want both of the square brackets to be considered when it does string interpolation you have to wrap the the array variable in curly braces {} like this:
<?php
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>{$contacts[0]['name']} : {$contacts[0]['email']}</li>\n";
echo "<li>{$contacts[1]['name']} : {$contacts[1]['email']}</li>\n";
echo "<li>{$contacts[2]['name']} : {$contacts[2]['email']}</li>\n";
echo "<li>{$contacts[3]['name']} : {$contacts[3]['email']}</li>\n";
echo "</ul>\n";
?>
The curly braces tells PHP exactly where the variable starts and ends which will work correctly.
Alternatively you can also use string concatenation (combining strings with .) instead of using string interpolation with double quotes.
Edit: Fixed some typos and cleaned up some sections.

james tarala
1,370 PointsThank you! That makes a lot of sense. Do you think there is a "best" out of the two examples? It seems the one you mentioned using the brackets is cleaner than adding a bunch of double-quotes.

andren
28,558 PointsNo, I don't really think either way is objectively better than the other. They have the same effect so it's mostly down to personal preference whether you prefer string concatenation or string interpolation. In cases where you are inserting a lot of variables it would indeed get a bit annoying constantly having to open and close strings, but it's not that rare a practice.
I tend to go for string interpolation whenever possible since I (as you) think it is cumbersome to combine many string together when you can keep all the content within one, especially since it makes spacing a lot easier to deal with as well. But that is a personal preference of mine, and there are certainly people who disagree.
When you work on your own you can go with whatever approach you prefer, though it's worth noting that if you end up joining a programming team at some point then It's pretty common to have a coding style guideline that dictates whether you use string concatenation or string interpolation and other such things. This is to ensure that the code the team writes is consistent and easy for the others to read. So in that case you may not have a choice either way.
james tarala
1,370 Pointsjames tarala
1,370 PointsThis guy: https://teamtreehouse.com/community/error-bummer-i-do-not-see-the-required-output-you-should-not-be-modifying-the-output-at-this-point, answered my question too! Thanks!