Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial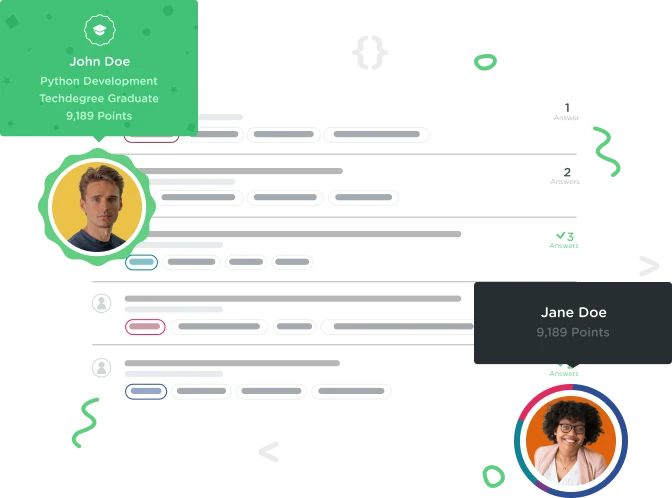

suheyb becerek
1,753 Pointsmultiple players showing but not moving
import random
import os
#draw grid
#pick random location for player
#pick random location for exit door
#pick random location for the monster
#draw player in the grid
#take input for the move
#move player unless invalid move
#check for w/l
#clear screen redraw grid
CELLS = [(0,0),(1,0),(2,0),(3,0),(4,0),
(0,1),(1,1),(2,1),(3,1),(4,1),
(0,2),(1,2),(2,2),(3,2),(4,2),
(0,3),(1,3),(2,3),(3,3),(4,3),
(0,4),(1,4),(2,4),(3,4),(4,4)]
def get_locations():
return random.sample(CELLS, 3)
def move_player(player,move):
x, y = player
#get player's location
#if move == LEFT, x-1
#if move == RIGHT,x+1
#if move == DOWN, y+1
#if move == UP , y-1
if move == "LEFT":
x -= 1
if move == "RIGHT":
x += 1
if move == "DOWN":
y += 1
if move == "UP":
y -= 1
return x, y
def get_moves(player):
moves = ["LEFT","RIGHT","UP","DOWN"]
x, y = player # unpacking player tuple to x and y
#if player's y == 0 they can't up
#if player's y == 4 they can't down
#if player's x == 0 they can't left
#if player's y == 4 they can't right
if x == 0:
moves.remove("LEFT")
if x == 4:
moves.remove("RIGHT")
if y == 0:
moves.remove("UP")
if y == 4:
moves.remove("DOWN")
return moves
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
def win_lose():
if player == monster:
print("monster: hahaa yummy dungeon master!!")
elif player == door:
print("You escaped from the dungeon !!")
def draw_map(player):
print(" _"*5)
tile = "|{}"
for cell in CELLS:
x ,y = cell
if x < 4:
line_end = ""
if cell == player:
output = tile.format("x")
else:
output = tile.format("_")
else:
line_end = "\n"
if cell == player:
output = tile.format("x|")
else:
output = tile.format("x|")
print(output,end=line_end)
def game_loop():
monster, door, player = get_locations()
while True:
draw_map(player)
valid_moves_player=get_moves(player)
print("You're currently in room {}".format(player)) #fill with player position
print("You can move {}".format(", ".join(get_moves(player)))) #fill with available moves
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in valid_moves_player:
player = move_player(player,move)
else:
input("\n walls are hard don't run into them!\n")
clear_screen()
clear_screen()
print("welcome to the dungeon")
input("Press return to start!!")
clear_screen()
game_loop()
_ _ _ _ _
|_|_|_|_|x|
|_|_|_|_|x|
|_|_|_|_|x|
|_|x|_|_|x|
|_|_|_|_|x|
You're currently in room (1, 3)
You can move LEFT, RIGHT, UP, DOWN
Enter QUIT to quit
as i said multiple players are showing in the right side of map when i move the player only one of them moves
Steven Seebode
2,631 PointsSteven Seebode
2,631 Pointsin your draw_map function, you have:
else: output = tile.format("x|") but should be else: output = tile.format("_|")
This is why you are seeing multiple x's