Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial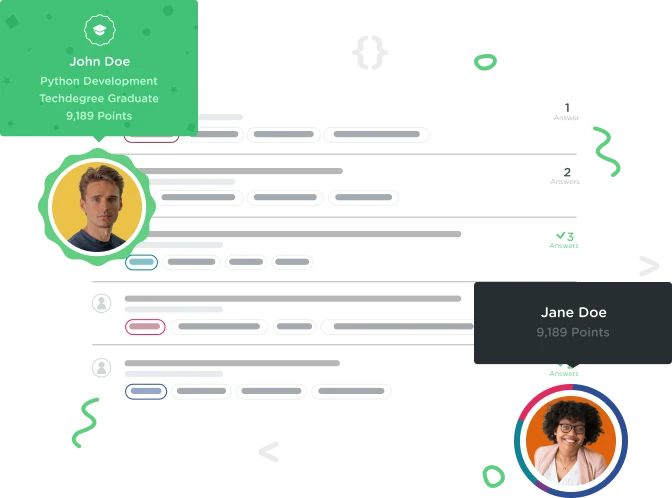

Ben Bushell
10,838 Pointsmultiple records with same name & record not found solution - would appreciate any feedback.
Hi,
Here are my solutions to the extra tasks. Took me a while to work out but got there in the end I think. Any feedback on the solution would be appreciated:
var students = [
{name: 'Ben', track: 'IOS', achievements: 10, points: 100},
{name: 'Vicky', track: 'JAVA', achievements: 10, points: 100},
{name: 'Ben', track: 'CSS', achievements: 15, points: 150},
{name: 'Dave', track: 'HTML', achievements: 20, points: 200},
{name: 'Tim', track: 'Android', achievements: 50, points: 500}
];
var message = "";
var search;
var found;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Enter a name to search or type 'quit' to exit");
found = false; //set found to false every time the prompt is called so previous true values do not change the result
if (search === null || search.toUpperCase() === 'QUIT') {
break;
}
for (i = 0; i < students.length; i += 1) {
student = students[i];
if (search.toUpperCase() === student.name.toUpperCase()) {
message += getStudentReport(student); //If more than one record with the same name will both be added to the message
print(message);
found = true;
}
}
console.log(found); //used this to help me test
if (!found) { //if not found alert will show
alert('Sorry ' + search + ' not found, please try again');
}
};
1 Answer

Thomas Grimes
10,726 PointsThanks for posting, Ben. I was going down a different path, trying to add a for loop within the student/search if statement. Your solution is definitely simpler than what I was trying. Here is my final code:
var message = '';
var student;
var search;
var found;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
found = false;//assume student value entered is not found
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
message += getStudentReport(student);
print(message);
found = true;
}
}
//if value is false alert user student is not found.
if(!found){
alert('Student not found!');
}
}