Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial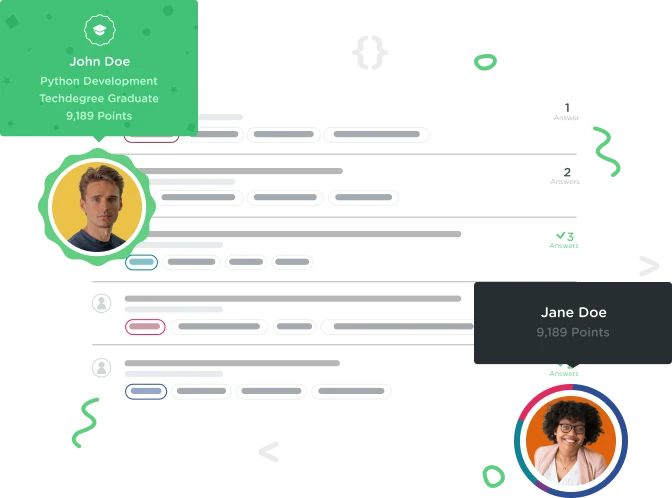

Jacob TRAN
653 PointsMultiple return keyword in a function
Why does the function use multiple return keyword when it only returns one output? What is an example of a function where it needed multiple return keyword in its code block?
3 Answers
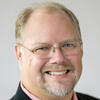
Jason Larson
8,361 PointsIt is quite common to have conditional statements in a function that have different return values. One benefit of this approach is that you can exit a function early and prevent having to process the rest of the statements in the function. For example, you might have a function like the following, which is from a blackjack game I coded, where I return early if neither the player nor the dealer have blackjack, but continue to do other things in the function if at least one of them has blackjack:
function checkForBlackJack() {
if (!dealer.hasBlackjack && !player.hasBlackjack) return false;
if (dealer.hasBlackjack) {
if (!player.hasBlackjack) result('lose', "Lose: (D:BJ)");
else result('push', "Push: (BJ)");
}
else {
result('bj', "Win! Blackjack!")
}
displayCards(player, cardsEl);
checkDealerHand();
return true;
}
Hopefully this clears things up for you, but if not, reply and I'll see if I can clarify.

Jacob TRAN
653 PointsI can see this from a conditional outcome, does this apply for conditionals only or are there any other cases where there are multiple returns? And yes this makes sense. Thank you
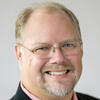
Jason Larson
8,361 PointsI can't think of how you could have more than one useful return without a conditional to force one to be executed vs. the other. You can of course put multiple returns in a function, but without some sort of condition to bypass the first one, the later ones will never get called because the function is exited when the return statement is called.