Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial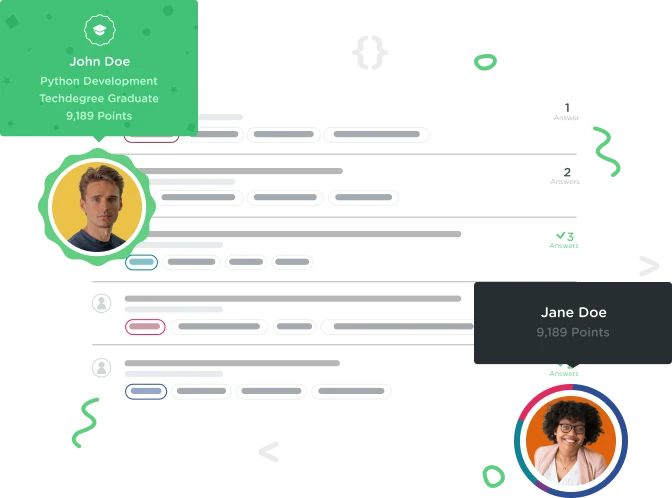

Unsubscribed User
3,052 PointsMultiple Values in return
Hello,
Great video but I am stumped by what I saw Dave do in this video. Specifically, I saw him add a couple of strings in the return keyword, which I thought was prohibited. In my code, which worked identical to his, I wrote (in the var):
function getArea(width, length, unit) { var area = (width * length) + " " + unit; return area; }
alert(getArea(10, 20, "ft.sq"));
However, he wrote (in the return):
function getArea(width, length, unit) { var area = (width * length) return area + " " + unit; }
alert(getArea(10, 20, "ft.sq"));
Both works fine, so could someone please elaborate how he is able to put a string and an argument into the return code? Are those not each individual values?
Thanks!
2 Answers
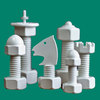
Steven Parker
231,268 PointsBoth versions are returning a single string. The plus signs are not performing math but string concatenation. The difference is that in your version, you perform the concatenation as you assign the string to the variable "area", which you return. And you need those parentheses to be sure the math calculation happens before any string conversion.
In the other version, the variable "area" is only assigned the result of the calculation, so it is a number instead of a string. But then in the return
statement, the same concatenation is performed to create a string (with no name) and that string is returned. His version doesn't actually have any parentheses (and does not need them).
So in both cases, what is returned is exactly the same thing.

Brandon Benefield
7,739 PointsSteven Parker has a great answer but I feel it may still leave you confused. The easiest way I can explain it, is to think about the return
statement just like an alert()
or console.log()
. In alert()
and console.log()
you can return anything you want as long as it is valid JavaScript. The only difference is a return
statement is used inside of a function
.
func sentence(greeting, goodbye) {
return greeting + ' ' + 'It was nice to see you!' + ' ' + goodbye;
}
sentence('Hello', 'See you later!') //returns 'Hello It was nice to see you! See you later!'
Is the equivalent of a simple console.log()
var greeting = 'Hello';
var goodbye = 'See you later!';
console.log(greeting + ' ' + 'It was nice to see you!' + ' ' + goodbye) //returns 'Hello It was nice to see you! See you later!'
Another example to help solidify this knowledge is attempting to use a return
statement outside of a function, you will be met with an error Uncaught SyntaxError: Illegal return statement
.
if (true === true) {
return true; // Uncaught SyntaxError: Illegal return statement
}
if (true === true) {
console.log(true); // returns 'true';
}

Unsubscribed User
3,052 PointsHi, thanks for the clarification. I think that makes sense now, but I will make sure to refer to this often as I continuously work on JS. Maybe I answered my own question, but it seems that in both instances, function or var, I "technicall" only put in one value, since concatenation is simply joining something to still make only ONE value? Is this correct to assume?

Brandon Benefield
7,739 PointsNick Caligari I never looked at it like that before but yea, I would say that is a great way to look at it.
Unsubscribed User
3,052 PointsUnsubscribed User
3,052 PointsHi Steven,
Thanks for getting back to me so quickly. Appreciate the answer and that makes sense, especially that you brought up concatenation.
Nick