Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial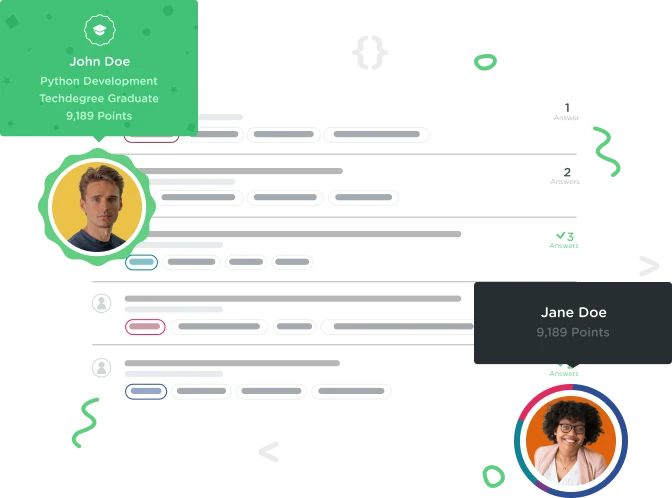

German Espitia
4,578 PointsMVC framework and Parse
Hello all, I wrote this question on SA and was hoping I could get some help from the treehouse forum as well.
In my app I have a TableViewController
which controls all the Habit
objects. So when they come in from Parse, I take each PFObject
and make a new Habit
object from it with their respective properties (name, current streak, date created, user who created it). After loading, the user might delete, edit, add new habits which leaves me question how I should handle all these modifications?
Should I keep going with my Habit
objects and with every modification upload the changes to Parse or simple leave the Habit
object out of it and deal with PFObject
s which in turn leaves me to write less code for each modification.
An example:
When the user creates a new Habit, I can either create the new Habit
object and the PFObject
as follows:
Habit *habit = [Habit habitWithTitle:self.habitField.text];
habit.frequency = self.howOften;
habit.currentStreak = @0;
// add it to back end
PFObject *newHabit = [PFObject objectWithClassName:@"Habit"];
newHabit[@"name"] = self.habitField.text;
newHabit[@"currentStreak"] = @0;
or forget about Habit
class and use PFObject
s and pass those around:
PFObject *newHabit = [PFObject objectWithClassName:@"Habit"];
newHabit[@"name"] = self.habitField.text;
newHabit[@"frequency"] = self.howOften;
newHabit[@"currentStreak"] = @0;
Another example would be when deleting a habit from a tableViewCell
swipe, I have to create the PFObject
from the Habit
object in order to send it to the back end, find it and delete it.
Where MVC comes in:
It is my understanding that in this situation, the Habit
object is the Model, the View is well the views and the controller is my class that manages the interaction between those two. Could my model be the PFObjects?
3 Answers
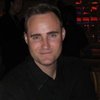
Dennis Walsh
5,793 PointsGerman,
If you are going to use Parse, I would suggest that you use the PFObjects that Parse provides rather then trying to maintain data in two differnet places. Creating your own objects will just cause you more work and syncronization issues as you mentioned in your post. Your model is the PFObjects which are just stored externally rather then on the device.
Parse provides a lot of support for this functionality, so be sure to read the documentation on creating/updating/deleting objects. Also, make sure to use the background processes when accessing data from Parse to keep the UI responsive. This can be challenging in that the processes are asynchronous so you do not know exactly when the task will be completed.

German Espitia
4,578 PointsThank you Dennis. I just wanted to make sure that it was OK to use PFObjects as my 'model'. Being a beginner makes me doubt myself.
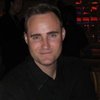
Dennis Walsh
5,793 PointsGerman,
Questioning is a good practice, I am not an expert by any means. The MVC pattern does not specify where the model data is stored, just that you keep the view, the model, and the controller independent of each other. This allows each component to function without knowing the details of how the other components are implemented.
There are no absolute answers when using any pattern (MVC is one of many patterns), they are guidelines on how others have successfully solved similar problems while keeping the code encapsulated, flexible and extensible. There are always exceptions, you just need a really good reason for any exception, and your specific case my require some "alterations" to adapt the pattern. You an also checkout these texts on the subject of design patterns if you want to learn more:
Design Patterns Explained: A New Perspective on Object-Oriented Design
Design Patterns: Elements of Reusable Object-Oriented Software

German Espitia
4,578 PointsDennis thank you for that information. I appreciate it!