Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial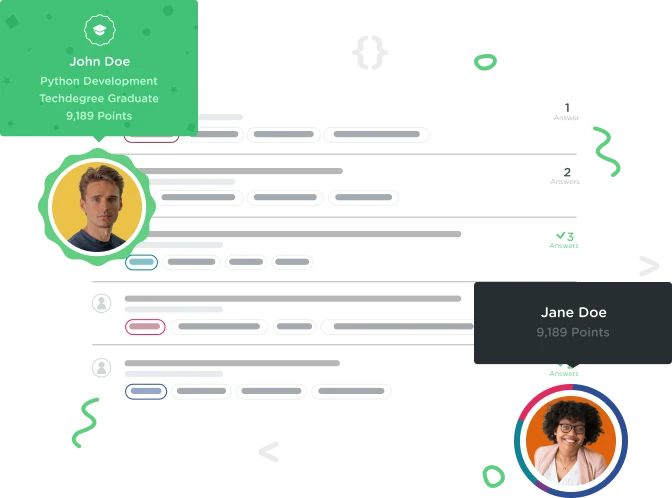

Lukas Baumgartner
14,817 PointsMVC-Pattern
Hey, I'd like to practice the MVC-Pattern and therefore I'm coding a simple TicTacToe app.
Now I just started and bumped into the first Question: Does a getter in my model conform with the MVC-Pattern? Am I allowed to do it or do I have to do it differently to apply to the rules of this pattern?
Here's my code so far:
model:
public class GameBoard {
public static final int SIZE = 3;
public static final int TURNS = SIZE * SIZE;
private Stone grid[][] = new Stone[SIZE][SIZE];
//Fill the new GameBoard with NONE(" ") Stones
public GameBoard(){
for(int r = 0; r < SIZE; r++){
for(int c = 0; c < SIZE; c++){
grid[r][c] = Stone.NONE;
}
}
}
//is this allowed?
public Stone getStone(int row, int col) {
return grid[row][col];
}
}
public enum Stone {
X("X"), O("O"), NONE(" ");
private final String stone;
Stone(String stone){
this.stone = stone;
}
@Override
public String toString() {
return stone;
}
}
view:
public class GameFieldView {
public void printGameField(GameBoard board){
System.out.println(" 1|2|3|");
for(int row = 0; row < GameBoard.SIZE; row++){
System.out.print(row);
for(int col = 0; col < GameBoard.SIZE; col++){
System.out.print("|");
System.out.print(board.getStone(row, col)); //is this a problem?
}
System.out.println("|");
}
}
}
controller:
public class GameController {
public void play(GameBoard model, GameFieldView view) {
view.printGameField(model);
}
}