Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial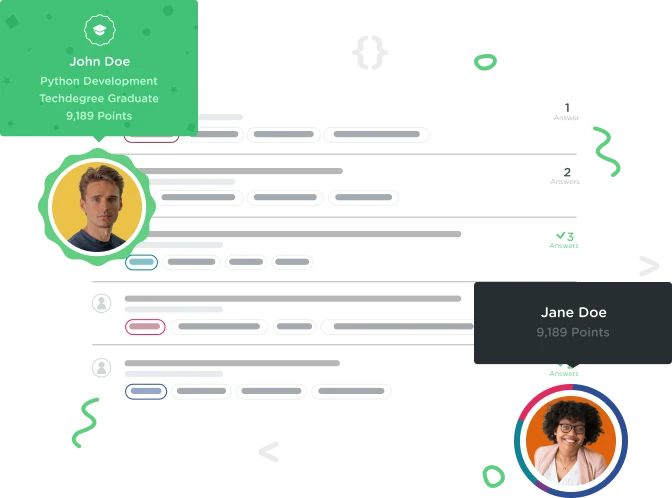

Harcharan Riyait
1,625 PointsMy 2 solutions using a Do While and For loops.
Here is my initial take on the question:
var colorCounter = 0;
function colorGen(){
var red = Math.floor(Math.random() * 256 );
var green = Math.floor(Math.random() * 256 );
var blue = Math.floor(Math.random() * 256 );
var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
var html = '<div style="background-color:' + rgbColor + '"></div>';
document.write(html);
colorCounter ++;
}
do {
colorGen();
} while (colorCounter <= 25);
Here is my second take on it:
for (i = 0; i < 10; i += 1){
var red = Math.floor(Math.random() * 256 );
var green = Math.floor(Math.random() * 256 );
var blue = Math.floor(Math.random() * 256 );
var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
var html = '<div style="background-color:' + rgbColor + '"></div>';
document.write(html);
}
I prefer the For loop but I keep coming back to the three var's for color generation. I think it could be shorter or more elegant. Any suggestions?
Also, how do I separate script from regular text in these posts? -Edit: Sorted by Chyno Deluxe. Thanks.
Thanks
4 Answers

Sascha Bratton
3,671 PointsHow about like this?
for (i = 0; i < 10; i++) {
var html = '<div style="background-color: ' + rgbColor() + ';"></div>';
document.write(html);
}
function rand256() { return Math.floor(Math.random() * 256 ); }
function rgbColor() { return 'rgb(' + rand256() + ',' + rand256() + ',' + rand256() + ')'; }

Harcharan Riyait
1,625 PointsThanks Sascha. That makes sense and looks really tidy.

Haley Elder
4,705 PointsAwesome! I like the "for" loop version, much simpler and I keep forgetting you can use the "i" for the loops, I was close the first time and just had to replace that basically :)

Allen Liff
5,003 PointsSascha: I like is the way the function rgbColor is laid out. It is intuitively obvious. Thus, another programmer, at a glance, can figure out what is going on.
Chyno Deluxe
16,936 PointsChyno Deluxe
16,936 PointsFixed: Code presentation.