Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial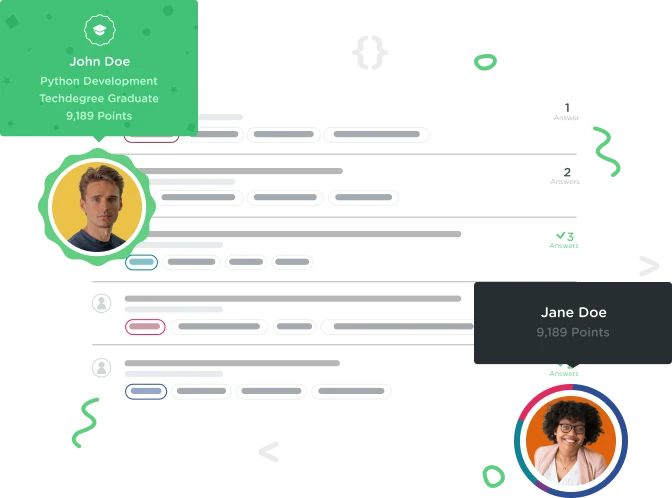

Marco Ricci
Front End Web Development Techdegree Graduate 23,880 PointsMy alternative solution without typing HTML
const ul = document.createElement("ul"); ul.className = "bulleted";
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () { if (xhr.readyState === 4) { var employeesList = JSON.parse(xhr.responseText);
employeesList.forEach((employee) => {
const li = document.createElement("li");
li.className = employee.inoffice ? "in" : "out";
li.textContent = employee.name;
ul.appendChild(li);
});
document.getElementById("employeeList").appendChild(ul);
} };
xhr.open("GET", "data/employees.json"); xhr.send();
4 Answers
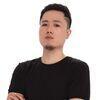
Billy Gragasin
Full Stack JavaScript Techdegree Student 4,985 PointsThere's an error since the "ul" element is not yet created. JavaScript returns a "null" error when appending it to the parent div element. Or I just did something wrong.
Anyway, the command for setting the class name is pretty neat! No more ugly if statement(s)!!

Daniel Ahn
Front End Web Development Techdegree Student 8,575 PointsYou have to move the <script src="js/widget.js"></script> down from the <head> section to right above the closing </body> tag. This will fix the error Billy is referring to. Wow...Treehouse really needs to update this particular course :P

Sarai Vasquez Mariñez
3,891 PointsI considered doing something similar but instead did the following which I guess is more apt for folks that are still uncomfortable with ternary operators:
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
let employees = JSON.parse(xhr.responseText);
let ul = document.createElement('ul');
document.getElementById('employeeList').insertAdjacentHTML('afterbegin', '<ul class="bulleted">');
for(let i = 0; i<employees.length; i++){
let employee = document.createElement('li');
employee.innerHTML = `${employees[i].name}`;
if(employees[i].inoffice === true){
employee.classList.add('in');
} else {
employee.classList.add('out');
};
document.querySelector('.bulleted').appendChild(employee);
}
}
};
xhr.open('GET', 'data/employees.json');
xhr.send();
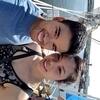
Andre Hammons
9,278 PointsNice! My solution turned out very similar. I just separated the status determination into a separate function.
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
// Select appropriate element to insert list.
const aside = document.getElementById('employeeList');
// Create the list and append.
const employeeList = createList(employees);
aside.appendChild(employeeList);
};
};
xhr.open('GET', 'data/employees.json');
xhr.send();
function getStatus(employee) {
// Returns 'in' or 'out' based on 'inoffice' property.
// Return value assigned to li class attribute.
if (employee.inoffice === true) {
return 'in';
} else {
return 'out';
};
};
function createList(employees) {
// Create unordered list.
const ul = document.createElement('ul')
// Loop through parsed JSON.
for (let employee of employees) {
// Create list item.
const li = document.createElement('li')
li.textContent = employee.name;
li.className = getStatus(employee);
ul.appendChild(li);
};
// Assign ul class attribute and return element.
ul.className = 'bulleted';
return ul;
};