Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial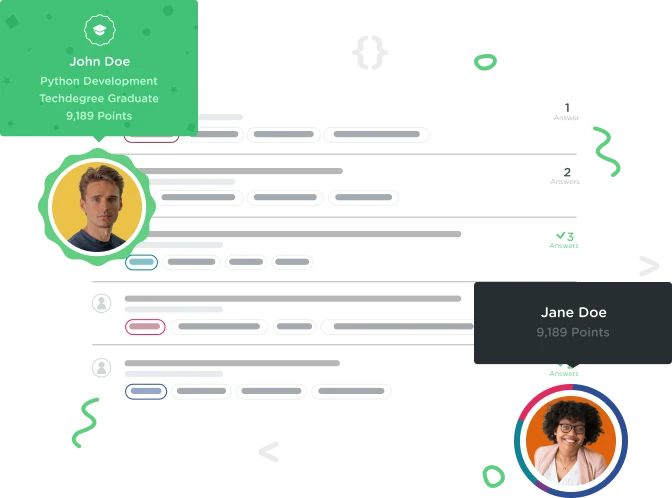

Michael Nanni
7,358 PointsMy answer to the challenge. Let me know what you think.
I decided to use a CTE with a few joins.
-- This CTE allows you to pull any class schedule for a student_id
WITH all_schedules AS (
SELECT * FROM CLASSES
JOIN SCHEDULE ON CLASSES.ID = SCHEDULE.CLASS_ID
JOIN SUBJECTS ON SUBJECTS.ID = CLASSES.SUBJECT_ID
JOIN PERIODS ON PERIODS.ID = CLASSES.PERIOD_ID
)
SELECT STUDENTS.FIRST_NAME, STUDENTS.LAST_NAME, PERIOD_ID, NAME, START_TIME, DURATION, ROOM_ID, TEACHERS.LAST_NAME
FROM all_schedules
JOIN TEACHERS ON TEACHERS.ID = all_schedules.TEACHER_ID
JOIN STUDENTS ON STUDENTS.ID = all_schedules.STUDENT_ID
WHERE STUDENTS.FIRST_NAME = 'Rex'
ORDER BY PERIOD_ID;
I thought a schedule should have any information that would be relevant to the student. They need to know where to go, what time to be there, how long the class it, and who teaches. The reason I did not join teachers and students in the CTE is because they both have first and last names, which would make them ambiguous when selecting them in the query later.
1 Answer
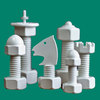
Steven Parker
231,269 PointsYou could also use column aliases to make them unambiguous and not need a CTE:
SELECT STUDENTS.FIRST_NAME,
STUDENTS.LAST_NAME,
PERIOD_ID as "PERIOD",
NAME as "CLASS",
START_TIME,
DURATION,
ROOM_ID as "ROOM",
TEACHERS.LAST_NAME as "TEACHER"
FROM CLASSES
JOIN SCHEDULE ON CLASSES.ID = SCHEDULE.CLASS_ID
JOIN SUBJECTS ON SUBJECTS.ID = CLASSES.SUBJECT_ID
JOIN PERIODS ON PERIODS.ID = CLASSES.PERIOD_ID
JOIN TEACHERS ON TEACHERS.ID = CLASSES.TEACHER_ID
JOIN STUDENTS ON STUDENTS.ID = SCHEDULE.STUDENT_ID
WHERE STUDENTS.FIRST_NAME = 'Rex'
ORDER BY PERIOD_ID;
For the benefit of other readers, could you post a link to the course page you were working with?