Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial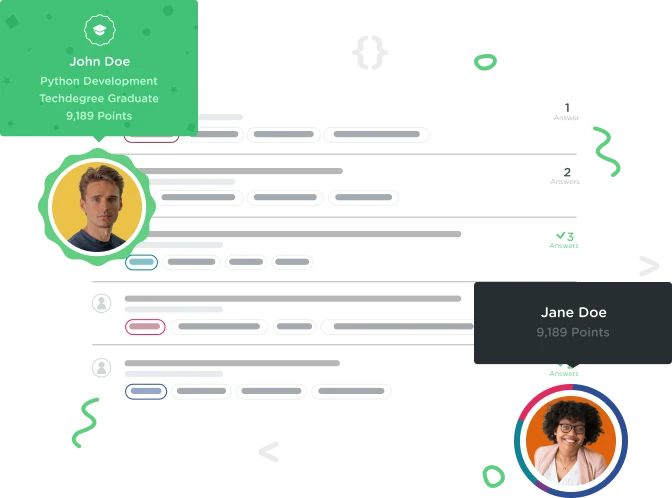
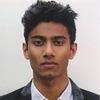
Samuel Kleos
Front End Web Development Techdegree Student 13,728 PointsMy answers are always wrong.
I just keep getting the prompt "Sorry that's incorrect. Moving on..." even if I answer with the correct answer.
// 1. Create a multidimensional array to hold quiz questions and answers
let questions = [
["How many stars in the galaxy", 1],
["What's my mums name?", "frank"],
["How much of a dum dum are you out of 10?", 10]
];
// 2. Store the number of questions answered correctly
let correct_answers;
/*
3. Use a loop to cycle through each question
- Present each question to the user
- Compare the user's response to answer in the array
- If the response matches the answer, the number of correctly
answered questions increments by 1
*/
for ( let i = 0; i < questions.length; i++ ) {
let guess = prompt(`Hi there, ${questions[i][0]}`);
let answer = questions[i][1]
if ( guess === answer ) {
alert("Correctamundo!");
correct_answers++;
} else {
alert("Sorry that's incorrect. Moving on...");
}
}
1 Answer
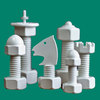
Steven Parker
241,808 PointsIf you tried the 2nd question, you would have found that it worked, though the other 2 did not. That's because guess is always a string, but on those questions answer is a number. And the type-sensitive comparison operator ( === ) will always consider items of different types to not match, no matter what the contents.
This is easily resolved by either storing the answers as strings in the array, or by using the standard comparison operator ( == ) instead.