Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial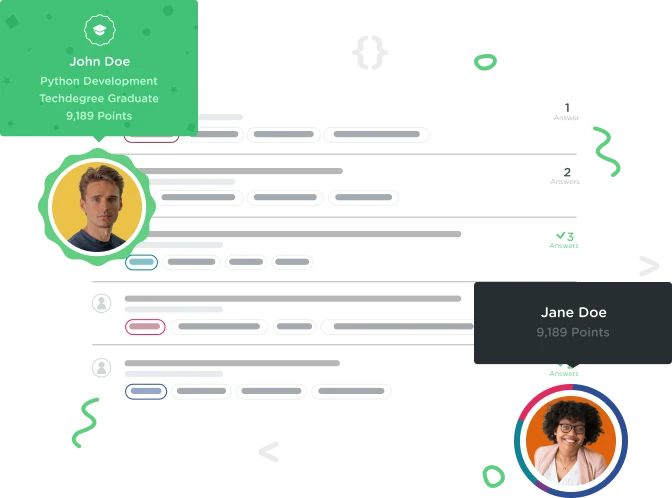

markwild
1,986 PointsMy answers come up wrong, even when typed correctly, what is happening here?
var correct;
var wrong;
var me = [
['What is my name?', 'Mike'],
['What is my age?', 20],
['What is my hobby?', 'coding']
]
for (var i = 0; i < me.length; i ++) {
if (prompt(me[i][0]).toLowerCase() === me[i][1]) {
console.log('You got ' + me[i][0] + ' right!');
correct ++;
} else {
console.log('Sorry, you got ' + me[i][0] + ' incorrect! :(');
wrong ++;
}
};
console.log( 'Correct: ' + correct + '&' + 'Wrong: ' + wrong)
1 Answer

Ross King
20,704 PointsHi markwild ,
You're on the right track so keep up the good work, there were just a few areas that need to be fixed.
Correct and Wrong Counts
When you create empty variables they are saved as "undefined". This caused a NaN error when trying to increment the correct and wrong answers.
To fix this you just need to set the counters to 0.
Answers
The answer to What is my name? was Mike, however in your conditional statements you converted the input from the user to lowercase and mike is not equal to Mike. To fix this I have changed the answer to lowercase.
The answer to What is my age? was the number 20, however, when you get data from a prompt it is always returned as a string, so the number 20 is not equal to "20". I have changed your answer to "20".
Code Example:
var correct = 0;
var wrong = 0;
var me = [
['What is my name?', 'mike'], // Changed Mike to mike (lowercase)
['What is my age?', "20"], // When getting information from prompts, the results are always strings and not numbers.
['What is my hobby?', 'coding']
];
for (var i = 0; i < me.length; i ++) {
// You are converting the answers to lowercase, however in the me array your answer to name was "Mike".
if (prompt(me[i][0]).toLowerCase() === me[i][1]) {
console.log('You got ' + me[i][0] + ' right!');
correct ++;
} else {
console.log('Sorry, you got ' + me[i][0] + ' incorrect! :(');
wrong ++;
}
};
// Added spaces around " & "
console.log( 'Correct: ' + correct + ' & ' + 'Wrong: ' + wrong);
There are other concepts that will clean up this code but the TreeHouse tutorials do a great job of explaining it as you progress, so don't worry if it doesn't make sense now. It will soon!
Good luck!
markwild
1,986 Pointsmarkwild
1,986 PointsThank you so much!