Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial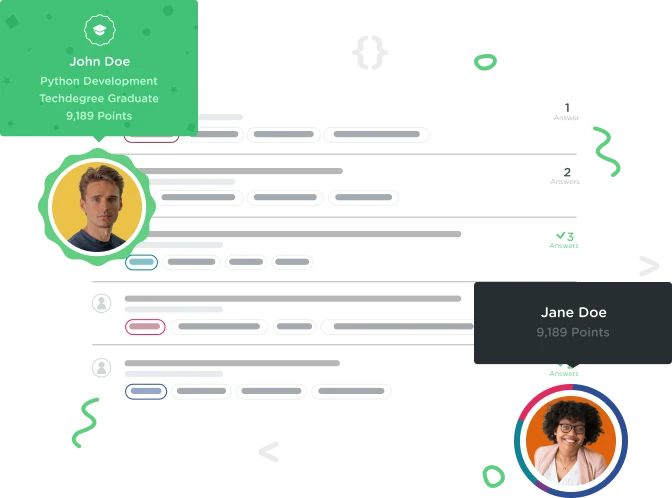
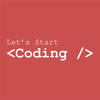
Samuel Llibre-Pillco
15,467 PointsMy approach on this project.
The HTML
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Arrays</title>
<link href="css/style.css" rel="stylesheet">
</head>
<body>
<main>
<h1>You got <span class="js-correct-score"></span> question(s) correct</h1>
<h2>You got these questions right:</h2>
<ol class="js-correct-answers">
</ol>
<h2>You got these questions wrong:</h2>
<ol class="js-wrong-answers"></ol>
</main>
<script src="js/quiz.js"></script>
</body>
</html>
The JavaScript
let questions = [
['How many planets are in the Solar System?', '8'],
['How many continents are there?', '7'],
['How many legs does an insect have?', '6'],
['What year was JavaScript created?', '1995']
];
let correctAnswer = 0;
for(let i = 0; i < questions.length; i++){
let answer = prompt(questions[i][0]);
if (answer === questions[i][1]) {
correctAnswer += 1;
document.querySelector(".js-correct-answers").innerHTML += `<li> ${questions[i][0]}`;
} else {
document.querySelector(".js-wrong-answers").innerHTML += `<li> ${questions[i][0]}`;
}
document.querySelector(".js-correct-score").textContent = correctAnswer;
}
I can think of 2 or 3 other option to do the same, but I found this the easiest and fastest to write.
1 Answer

Emily Humphrey
5,404 PointsYou don't have to, but you should always close your element tags. It's best practice to do so as it increases readability and maintainability. Also, if you don't, they'll get popped in a validator or other monitoring tools like SEMRush.
Other than that, the code looks pretty ok! I really like your proper use of h1s and h2s. A few things to improve your code:
- Answer isn't going to change, so that should use the
const
keyword instead of let. - Instead of constantly referring to
questions[i][0]
, just set a const value at the top. This will increase readability and while this is minute, it's good practice. It requires more memory usage to look for a key in an array twice times than it does to do it once and then a locally scoped variable twice. -
document.querySelector
is an expensive operation. You have it in a loop, which means it's running 4 times each. It'll be more optimized, and readable, to put it in global scope as a const then reference it the loop. This takes your 12 dom operations and makes it 3. - For loop is fine, but it's a bit messy syntax. if your only goal is to loop over the array, it would be cleaner to utilize Array.forEach.
- This is more for formatting, but it's important. Take pride in your code. Be sure to use consistent formatting. Having sloppy HTML will get thrown back in a code review every single time and it's really just a lazy thing. Indent properly, have a cohesive strategies for linebreaks etc etc.
I refactored your JavaScript in the ways I mentioned above. Hope this helps!
let questions = [
['How many planets are in the Solar System?', '8'],
['How many continents are there?', '7'],
['How many legs does an insect have?', '6'],
['What year was JavaScript created?', '1995']
];
let correctAnswer = 0;
const $correctAnswers = document.querySelector(".js-correct-answers");
const $wrongAnswers = document.querySelector(".js-wrong-answers");
const $correctScore = document.querySelector(".js-correct-score");
questions.forEach(item => {
let answer = prompt(item[0]);
if (answer === item[1]) {
correctAnswer += 1;
$correctAnswers.innerHTML += `<li> ${item[0]}</li>`;
} else {
$wrongAnswers.innerHTML += `<li>${item[0]}</li>`;
}
$correctScore.textContent = correctAnswer;
})