Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial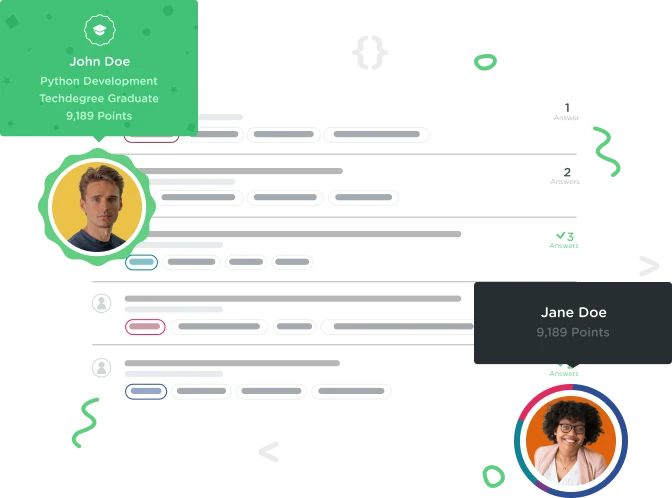

Timothy Smith
2,072 PointsMy attempt was a lot lighter. How can I improve answer testing?
I was surprised to see how much shorter my code was compared to Dave's. I ran into an issue when trying to add answer testing though.
var queA = [['What color is the sun?','red'],['What do dogs eat?','meat'],['Are penguins slick','yes']];
var score = 0;
function print(score) {
document.write('Congratulations, you scored ' + score + ' points!');
}
for (var i = 0; i < queA.length; i += 1) {
var answer = prompt(queA[i][0]);
if (answer === queA[i][1]) {
score += 1;
}
}
print(score);
Why can't I use '.toLowerCase' on the answer variable inside of the if statement?
2 Answers

Dan Johnson
40,532 Pointsif (answer.toLowerCase() === queA[i][1]) {
score += 1;
}
Works fine for me. Did you forget to add the parentheses when you tried?

Timothy Smith
2,072 PointsDoh! I completely forgot about the parentheses. Thanks for that.

MONICA BUI
3,526 PointsThis is a piece of your code:
for (var i = 0; i < queA.length; i += 1) {
var answer = prompt(queA[i][0]); //NOT EFFICIENT The browser need to create a variable again and again
if (answer === queA[i][1]) {
score += 1;
}
}
I suggest creating your variable OUTSIDE the loop, by doing this the browser doesn't have to create a variable every time it run through the loop, instead it just need to update the new value of the variable, like this:
var answer; // create out side the loop
for (var i = 0; i < queA.length; i += 1) {
answer = prompt(queA[i][0]); //every time the loop run the variable is updated with a new value
if (answer === queA[i][1]) {
score += 1;
}
}
This is how I wrote my code:
var questionAndAnswer = [
['A', 'A'],
['B', 'B'],
['C', 'C']
];
var correct = 0;
var wrong = 0;
var ask;
for (var i = 0; i < questionAndAnswer.length; i+=1) {
ask = prompt(questionAndAnswer[i][0]);
if (ask.toUpperCase() === questionAndAnswer[i][1]) {
correct = correct+=1;
} else {
wrong = wrong +=1;
}
}
function print(meg) {
document.write(meg)
}
print("<p> You've got " + correct + " correct answers </p>")
print("<p> You've got " + wrong + " wrong answers </p>")
Cheers! Monica
Timothy Smith
2,072 PointsTimothy Smith
2,072 PointsIn the next video I realized I was using the print function wrong as well. Here is my updated code: