Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial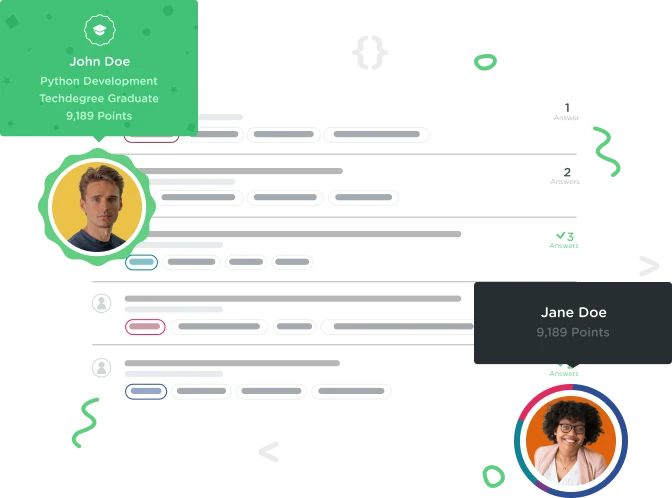
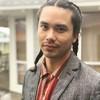
Jay Reyes
Python Web Development Techdegree Student 15,937 PointsMy attempt with localStorage
This was fun all the way to the dead end.
The biggest problem is that I can not access values inside the key. So all the individual Remove
and Edit
buttons are useless with localStorage. The true way to utilize them is with an actual database, I think.
Note I tried to use the text
parameter inside my removeInvitee
function to use as the value inside the invitees
string.
document.addEventListener('DOMContentLoaded', () => { const form = document.getElementById('registrar'); const input = form.querySelector('input'); const mainDiv = document.querySelector('.main'); const ul = document.getElementById('invitedList');
const div = document.createElement('div');
const filterLabel = document.createElement('label');
const filterCheckbox = document.createElement('input');
filterLabel.textContent = "Hide those who haven't responded";
filterCheckbox.type = 'checkbox';
div.appendChild(filterLabel);
filterLabel.appendChild(filterCheckbox);
mainDiv.insertBefore(div, ul);
filterCheckbox.addEventListener('change', (e) => {
const isChecked = e.target.checked;
const lis = ul.children;
if (isChecked) {
for (let i = 0; i < lis.length; i += 1) {
let li = lis[i];
if (li.className === 'responded') {
li.style.display = '';
} else {
li.style.display = 'none';
}
}
} else {
for (let i = 0; i < lis.length; i += 1) {
let li = lis[i];
li.style.display = '';
}
}
});
// LOCAL STORAGE
function supportsLocalStorage() {
try{
return 'localStorage' in window && window['localStorage'] !== null;
} catch(e) {
return false;
}
}
function getInvitees() {
var invitees = localStorage.getItem('invitees');
if(invitees) {
return JSON.parse(invitees);
} else {
return [];
}
}
function saveInviteeString(str) {
var invitees = getInvitees();
if(!str || invitees.indexOf(str) > -1) {
return false;
}
invitees.push(str);
localStorage.setItem('invitees', JSON.stringify(invitees));
return true;
}
function removeInvitee(text) {
localStorage.removeItem('invitees', text);
}
// creating the li node
function createLI(text) {
const li = document.createElement('li');
function createElement(elementName, property, value) {
const element = document.createElement(elementName);
element[property] = value;
return element;
}
function appendToLI(elementName, property, value) {
const element = createElement(elementName, property, value);
li.appendChild(element);
return element;
}
appendToLI('span', 'textContent', text);
appendToLI('label', 'textContent', 'Confirmed').appendChild(createElement('input', 'type', 'checkbox'));
appendToLI('button', 'textContent', 'edit');
appendToLI('button', 'textContent', 'remove');
return li;
}
if (supportsLocalStorage()) {
// Initialize display list with the invitees array
var invitees = getInvitees();
invitees.forEach( (inviteeString) => {
const li = createLI(inviteeString);
ul.appendChild(li);
});
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = '';
if (saveInviteeString(text)) {
const li = createLI(text);
ul.appendChild(li);
}
});
ul.addEventListener('change', (e) => {
const checkbox = e.target;
const checked = checkbox.checked; //checked is a boolean attribute
const listItem = checkbox.parentNode.parentNode;
if (checked) {
listItem.className = 'responded';
} else {
listItem.className = '';
}
});
ul.addEventListener('click', (e) => {
if (e.target.tagName === 'BUTTON') {
const button = e.target;
const li = e.target.parentNode;
const ul = li.parentNode;
const action = button.textContent;
const nameActions = {
remove: () => {
const span = li.firstElementChild;
removeInvitee(span.textContent)
ul.removeChild(li);
},
edit: () => {
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
input.value =span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'save';
},
save: () => {
const input = li.firstElementChild;
const span = document.createElement('span');
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
button.textContent = 'edit';
}
}
// select and run action in button's name
nameActions[action]();
}
});
}
});