Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial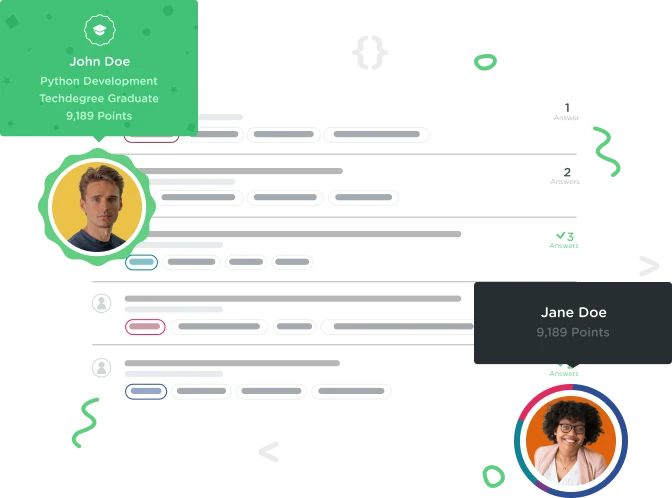

Bryce Edwards
1,586 PointsMy brain hurts. Can't figure this out, so bad at for loops!
Help please! I'm so bad!
var number = 4;
for (i = 1, i < 156, 1++) {
console.log(4 + i);
}
1 Answer

Joe Beltramo
Courses Plus Student 22,191 PointsYou have a few issues here.
First, the each section of thefor
loop should be treated as it's own statement. In JavaScript, a statement that lives on the same line as another statement must end in a semicolon:
for (var i = 4; i <= 156; i++) {
// code for loop
}
If you notice, in the example, each statement (with the exception of the last one) ends in a semicolon.
Second, when creating a variable within a loop, you should specify what type of variable it is with either the var
keyword. You can also declare the variable outside of the loop, though it is common to just use a var
in the for loop itself:
var i = 4;
for (i; i <= 156; i++) {
// code for loop
}
// or
for (var i = 4; i <= 156; i++) {
// code for loop
}
Third, we want to increment the value of i
which is where the i++
comes into play. Your example looks like a mistype using the number 1
instead of the variable i
. Look at the examples above to see.
Lastly, the challenge is looking for you to print the numbers 4 all the way to/and including the number 156. To do this, you will want to use <=
as in the examples above. Since the loop automatically increments the value for you, you simply need to log the value of the variable itself by doing this (inside the for
loop):
console.log(i);
Does that help?
Bryce Edwards
1,586 PointsBryce Edwards
1,586 PointsYou're the best. Thankyou!