Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial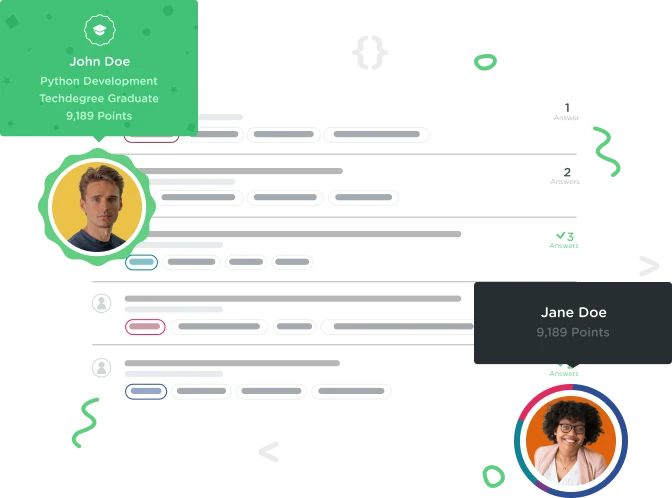
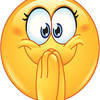
Bogdan Siverchuk
Courses Plus Student 1,707 PointsMY brain refused to work, seems simple but i can't get it, Map and Set how do they work together!?
Help!
package com.example.model;
import java.util.Map;
import java.util.Set;
import java.util.HashMap;
public class Contact {
private String mFirstName;
private String mLastName;
private Map<String, String> mContactMethods;
public Contact(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName;
/* This stores contact methods by name
* eg: "phone" => "(555) 555-1234"
*/
mContactMethods = new HashMap<String, String>();
}
public void addContactMethod(String method, String value) {
// TODO: Add to the contact method map
mContactMethods.put(method,value);
}
/**
* Returns the available contact methods. eg: phone, pager,
*
* @return The name of the contact methods that are available
*/
public Set<String> getAvailableContactMethods() {
// FIXME: This should return the current contact method names.
return null;
}
/**
* Returns the value for the contact method if it exists,
*
* @param methodName The name of the contact method to look up.
* @return The name of the contact methods that are available
*/
public String getContactInfo(String methodName) {
// FIXME: return the value for the passed in *methodName*
return null;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
4 Answers

Collin Halliday
Full Stack JavaScript Techdegree Student 17,491 PointsBogdan,
One thing that really helps me when I feel stuck is to review the documentation at the Oracle Help Center. I am constantly reviewing the documentation, even if its just to refresh my memory on a subject I have already covered. When I saw your question, the first thing I did was to type "java hashmap" into Google. The first search result was the Oracle Help Center's page on HashMaps. I opened that page, I clicked on the list of methods for that class, and I then began to read through the method descriptions until I got to keySet(). This method summary provides as follows: "Returns a Set view of the keys contained in this map." This sounds exactly like what we want in our getAvailableContactMethods() method. Indeed, you can simply return a call to that method on the mContactMethods HashMap.
For the third part of the challenge, I returned to the list of methods and their descriptions at the Oracle Help Center, and I read through each method description until I reached the get() method. This method summary provides as follows: "Returns the value to which the specified key is mapped, or null if this map contains no mapping for the key." So it takes a key as an argument and returns the associated value. This sounds like exactly what we want for the getContactInfo() method. Indeed, you can simply return a call to that method on the mContactMethods HashMap, passing the methodName parameter as an argument to the get() method.
I hope that is somewhat helpful. Best of luck!

Collin Halliday
Full Stack JavaScript Techdegree Student 17,491 PointsIt really just depends on the situation. It does not hurt to look at the documentation for both Set and HashMap. In the context of this problem, I chose to look at HashMap because that is the data structure into which our contact method data is stored and from which we wish to extract contact method data in our Contact Class. For the getAvailableContactMethods() method, we can see from the return type that we want the method to return a Set of data, in this case, the available contact methods that are stored as keys in our mContactMethods HashMap. The keySet() method provides a quick and direct solution to this problem. You won't always find a simple solution through one method call, but the documentation is a great place to start.
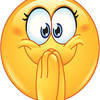
Bogdan Siverchuk
Courses Plus Student 1,707 PointsThank you!

Collin Halliday
Full Stack JavaScript Techdegree Student 17,491 PointsYou are very welcome.
Bogdan Siverchuk
Courses Plus Student 1,707 PointsBogdan Siverchuk
Courses Plus Student 1,707 Pointshow do you know what to look for ? i mean why java hashmap not just java set?