Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial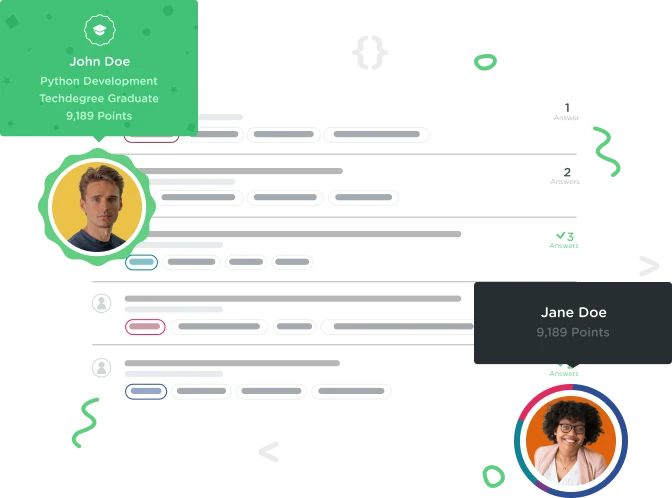
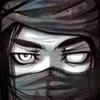
David Mendiola
Full Stack JavaScript Techdegree Student 16,579 PointsMy build a quiz challenge solution! Any extra pointers?
var answer = "",
answerCounter = 0,
correctAnswers = [],
wrongAnswers = [];
//Quiz questions
var quizQuestions = [
["What is the human body mostly made of?", "Water"],
["How many corners does a square have?", "4"],
["The greatest NBA franchise is what team?", "Lakers"]
];
//Determine correct / wrong answers
for (var x = 0; x < quizQuestions.length; x++){
answer = prompt(quizQuestions[x][0]);
answer = answer.toLowerCase();
quizQuestions[x][1] = quizQuestions[x][1].toLowerCase();
if (answer === quizQuestions[x][1]){
correctAnswers.push(quizQuestions[x][0]);
answerCounter += 1;
} else {
wrongAnswers.push(quizQuestions[x][0]);
}
}
//Function takes array to loop and print
function loopAnswers(finalAnswers){
for (var x = 0; x < finalAnswers.length; x++){
document.write("<li>" + finalAnswers[x] + "</li>");
}
}
//Quiz results
document.write("<h1>Pop Quiz</h1>");
document.write("<p>You answered " + answerCounter + " out of " + quizQuestions.length + " questions correctly.</p>");
if (correctAnswers.length > 0){
document.write("<h2>Questions you answered correctly:</h2>");
document.write("<ul>");
loopAnswers(correctAnswers);
document.write("</ul>");
}
if (wrongAnswers.length > 0){
document.write("<h2>Questions with wrong answers:</h2>");
document.write("<ul>");
loopAnswers(wrongAnswers);
document.write("</ul>");
}
1 Answer
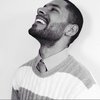
Michael Liendo
15,326 PointsNice work! A slight optimization would be to refactor your quizQuestions array to be an array of objects. That way your code could read quizQuestions[i].question.answer. It just makes it easier for a developer to understand what's going on. Also, it would allow you to create multiple answers for a question without having to remember the index ie: quizQuestions[i].question.answer[2]. Aside from that, the only other thing I'd suggest would be to refactor your bottom if-statements so that their a function since they're basically doing the same thing (DRY), but for a small quiz app like this, it's not such a big deal. Good job!
David Mendiola
Full Stack JavaScript Techdegree Student 16,579 PointsDavid Mendiola
Full Stack JavaScript Techdegree Student 16,579 PointsThanks! I complete refactored my code still no objects but alot of reusing.